Complete Tutorial On If And If Commands Else Commands In Java
In this tutorial, you will learn to use two selections of If Commands: if and if… else to control the program execution flow.
In programming, executing a particular code is often desirable based on whether the specified condition is true or false (determined only at runtime).
For such cases, control flow commands are used.
If Commands (if-then in Java)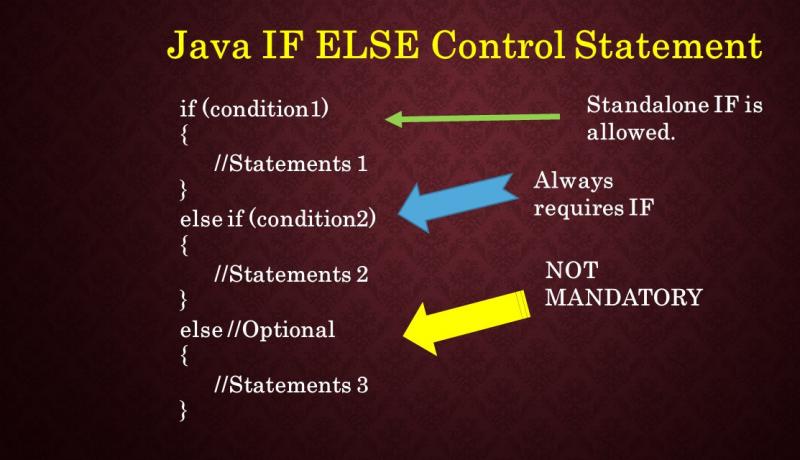
The syntax of the if-then Command in Java is as follows:
if (expression) { // statements }
Here, the expression is a Boolean expression (true or false).
If the expression is evaluated correctly, the statements inside the if body (in parentheses) are executed.
If the expression is incorrectly assessed, the statements inside the if body will not be executed.
How does the if statement work?
Example 1: If statement in Java
- class IfStatement {
- public static void main (String [] args) {
- int number = 10;
- if (number> 0) {
- System.out.println (“Number is positive.”);
- }
- System.out.println (“This statement is always executed.”);
- }
- }
Output
Number is positive.
This statement is always executed.
When the number equals 10, the expression> 0 is considered correct. Hence, the code inside the if body is executed.
Now, change the number value to a negative integer. For example -5. The output in this case will be equal to:
This statement is always executed.
When the number is equal to -5, the expression number> 0 is considered incorrect. Therefore, the Java compiler overrides the if body commands.
Command (if… else (if-then-else in Java)
A specific part of the code will be executed if the condition is evaluated correctly. The if statement may also have an else block. If the condition is incorrectly assessed, the commands in the else body are executed.
The syntax of the if-then-else Command is as follows:
if (expression) { // codes } else { // some other code }
How does the if… else Command work?
Example 2: The if… else Command in Java
- class IfElse {
- public static void main (String [] args) {
- int number = 10;
- if (number> 0) {
- System.out.println (“Number is positive.”);
- }
- else {
- System.out.println (“Number is not positive.”);
- }
- System.out.println (“This statement is always executed.”);
- }
- }
Output
Number is positive.
This statement is always executed.
When a number equals 10, the expression> 0 is evaluated correctly. In this case, the code inside the if body will be executed, and the code inside the else body will not be executed.
Now, change the value of the number to a negative number. For example -5. The output in this case will be equal to:
Number is not positive.
This statement is always executed.
When the number is -5, the expression> 0 is evaluated incorrectly. In this case, the code inside the else body will be executed, and the code inside the if body will not.
The Command if else… if in Java
In Java, executing one piece of code out of many codes is possible. You can use if… else… if to do this.
if (expression1) { // codes } else if (expression2) { // codes } else if (expression3) { // codes } . . else { // codes }
The if statements are executed from top to bottom. As soon as the condition is proper, the corresponding code is executed. After that, the program control jumps out of the if… else… if.
If all test statements are incorrect, the code inside the else body will be executed.
Example 3: If else… if statement in Java
- class Ladder {
- public static void main (String [] args) {
- int number = 0;
- if (number> 0) {
- System.out.println (“Number is positive.”);
- }
- else if (number <0) {
- System.out.println (“Number is negative.”);
- }
- else {
- System.out.println (“Number is 0.”);
- }
- }
- }
Output
Number is 0.
When a number is 0, both number> 0 and number <0 are considered incorrect. Hence, the code is executed inside another body.
The above program checks whether the number is positive, negative, or 0.
The if… else Command nested in Java
It is possible to have nested if..else commands in Java.
Here is a program to find the most significant number out of 3:
Example 4: If else nested Command
- class Number {
- public static void main (String [] args) {
- Double n1 = -1.0, n2 = 4.5, n3 = -5.3, largestNumber;
- if (n1> = n2) {
- if (n1> = n3) {
- largestNumber = n1;
- } else {
- largestNumber = n3;
- }
- } else {
- if (n2> = n3) {
- largestNumber = n2;
- } else {
- largestNumber = n3;
- }
- }
- System.out.println (“Largest number is” + largestNumber);
- }
- }
Output
Largest number is 4.5
Note: In the above programs, we have assigned values to the variables ourselves to make this easier. However, in real-world applications, these values may be derived from user input data, log files, submitted forms, etc.