Builders In Java – Complete Tutorial
In this tutorial, you will get acquainted with builders in Java and learn how to create and use builders with the help of examples.
What is a Builder?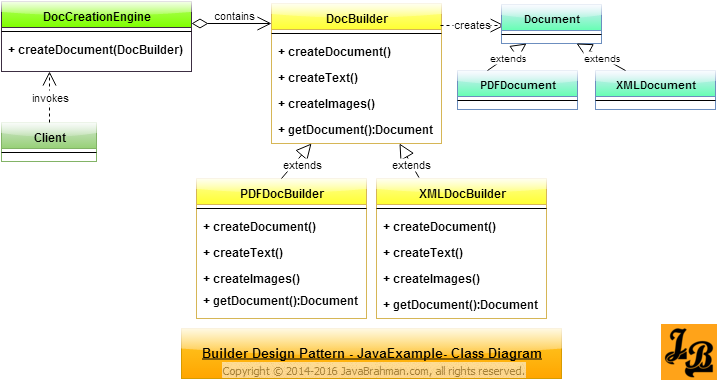
The constructor is similar to the method (but not the technique) that is called immediately when the object is created.
The Java compiler distinguishes between method and constructor using the name and recursive. In Java, the constructor has a class-like name and returns no values.
class Test { Test () { // constructor body } }
Here, Test () is constructive. It has the same class name and no return type.
class Test { void Test () { // method body } }
Here, Test () has the same name as the class. However, its return type is void. Hence, it is not constructive but methodical.
Example: Builder in Java
- class ConsMain {
- private int x;
- // constructor
- private ConsMain () {
- System.out.println (“Constructor Called”);
- x = 5;
- }
- public static void main (String [] args) {
- ConsMain obj = new ConsMain ();
- System.out.println (“Value of x =” + obj.x);
- }
- }
Output
Constructor Called
Value of x = 5
Here, the ConsMain () constructor is called when the obj object is created.
A constructor may or may not accept an argument.
Builder without arguments
If the Java constructor does not accept any parameters, the constructor is no-arg. Its structure is as follows:
accessModifier ClassName () { // constructor body }
Sample constructor without arguments
- class NoArgCtor {
- int i;
- // constructor with no parameter
- private NoArgCtor () {
- i = 5;
- System.out.println (“Object created and i =” + i);
- }
- public static void main (String [] args) {
- NoArgCtor obj = new NoArgCtor ();
- }
- }
Output
Object created and i = 5
Here, the NoArgCtor () constructor does not accept any parameters.
Did you notice that the creator of NoArgCtor () is private?
This is because the object is made from within the same class. Hence, it can access the manufacturer.
However, if the object is created outside of class, you must make access to that constructor public. For example:
- class Company {
- String domainName;
- // object is created in another class
- public Company () {
- domainName = “programiz.com”;
- }
- }
- public class CompanyImplementation {
- public static void main (String [] args) {
- Company companyObj = new Company ();
- System.out.println (“Domain name =” + companyObj.domainName);
- }
- }
Output
Domain name = programiz.com
Default builder
The Java compiler automatically creates an argument-free constructor at runtime if you do not make the constructor. This builder is known as the default builder. The default constructor initializes each of the informal sample variables.
Example: Default builder
- class DefaultConstructor {
- int a;
- boolean b;
- public static void main (String [] args) {
- DefaultConstructor obj = new DefaultConstructor ();
- System.out.println (“a =” + obj.a);
- System.out.println (“b =” + obj.b);
- }
- }
The above program is equivalent to:
- class DefaultConstructor {
- int a;
- boolean b;
- private DefaultConstructor () {
- a = 0;
- b = false;
- }
- public static void main (String [] args) {
- DefaultConstructor obj = new DefaultConstructor ();
- System.out.println (“a =” + obj.a);
- System.out.println (“b =” + obj.b);
- }
- }
Output
a = 0
b = false
Parametric constructor
A constructor may also accept parameters. Its structure is as follows:
accessModifier ClassName (arg1, arg2,…, argn) { // constructor body }
Example: Parametric constructor
- class Vehicle {
- int wheels;
- private Vehicle (int wheels) {
- wheels = wheels;
- System.out.println (wheels + ”wheeler vehicle created.”);
- }
- public static void main (String [] args) {
- Vehicle v1 = new Vehicle (2);
- Vehicle v2 = new Vehicle (3);
- Vehicle v3 = new Vehicle (4);
- }
- }
Output
2 wheeler vehicle created.
3 wheeler vehicle created.
4 wheeler vehicle created.
We passed an int (number of wheels) argument to the constructor when creating the object.
Overload builders in Java
Like method overload, you can have two or more manufacturers with the same name and parameters. For example:
- class Company {
- String domainName;
- public Company () {
- this.domainName = “default”;
- }
- public Company (String domainName) {
- this.domainName = domainName;
- }
- public void getName () {
- System.out.println (this.domainName);
- }
- public static void main (String [] args) {
- Company defaultObj = new Company ();
- Company programizObj = new Company (“programiz.com”);
- defaultObj.getName ();
- programizObj.getName ();
- }
- }
Output
default
programiz.com
important points
- Immediately after calling the objects, the creators are called.
- The two laws of creation are:
- The Java constructor name must match the class name exactly.
- A Java constructor should not have a recursive type.
- If the Java compiler does not have a constructor class, it automatically creates a default constructor at runtime. The default constructor initializes the sample variables with the default values. For example, the variable int is equal to 0.
- Manufacturer types:
- No-Arg Constructor – A constructor that accepts no arguments
- Default Builder – A builder created automatically by a Java compiler. (If not explicitly defined)
- Parametric constructor – Used to specify specific values of variables in an object
- Creators cannot be abstract or static or final.
- The constructor can be defined several times.