Lists In R: Lists Are Objects In R That Contain Elements Of Different Types
Lists in R: Lists are objects in R that contain elements of different types, such as numbers, strings, vectors, and other lists within them. A list can include a matrix or a function as its elements.
In R, Lists are created using the List () function.
Create Lists In R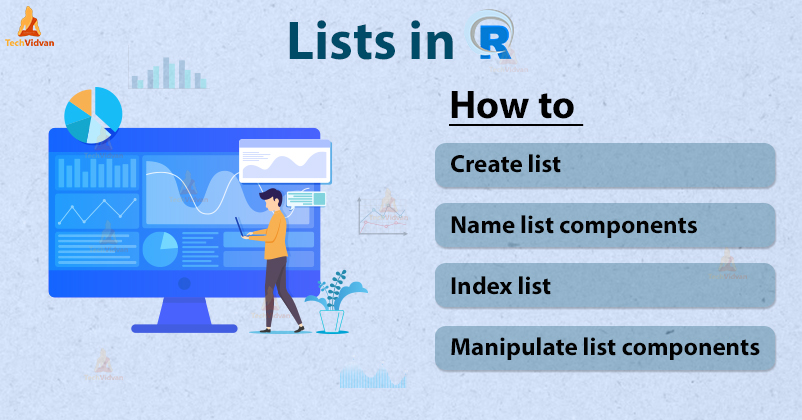
The following is an example of how to create a list of logical strings, numbers, and values.
# Create a list containing strings, numbers, vectors and a logical # values. list_data <- list (“Red”, “Green”, c (21,32,11), TRUE, 51.23, 119.1) print (list_data)
When we run the above code, the following results are generated:
[[1]] [1] “Red” [[2]] [1] “Green” [[3]] [1] 21 32 11 [[4]] [1] TRUE [[5]] [1] 51.23 [[6]] [1] 119.1
Name The Members of the List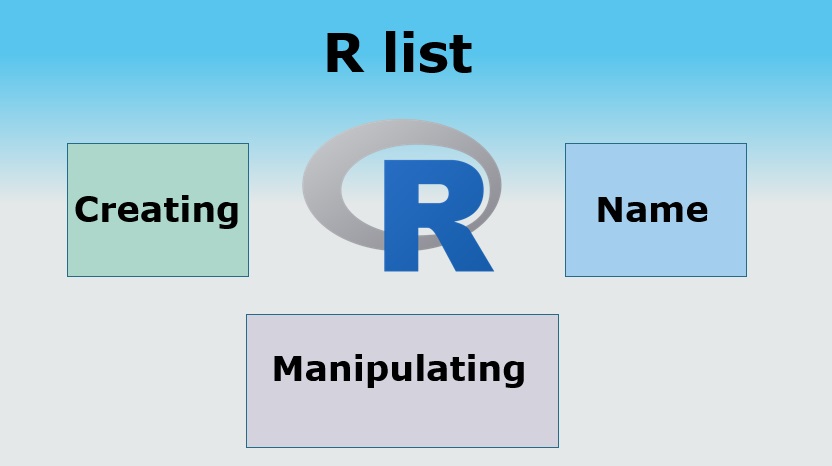
List members can be named and accessed using their names.
# Create a list containing a vector, a matrix and a list. list_data <- list (c ("Jan", "Feb", "Mar"), matrix (c (3,9,5,1, -2,8), nrow = 2), list (“green”, 12.3)) # Give names to the elements in the list. names (list_data) <- c (“1st Quarter”, “A_Matrix”, “A Inner list”) # Show the list. print (list_data)
When we run the above code, the following result is created:
$ `1st_Quarter` [1] “Jan” “Feb” “Mar” $ A_Matrix [, 1] [, 2] [, 3] [1,] 3 5 -2 [2,] 9 1 8 $ A_Inner_list $ A_Inner_list [[1]] [1] “green” $ A_Inner_list [[2]] [1] 12.3
Access the elements of a list
They can be accessed using the index of the List’s members. In the case of named lists, they can be accessed using the names of the Nir members.
We use the List in the example above; We continue:
# Create a list containing a vector, a matrix and a list. list_data <- list (c ("Jan", "Feb", "Mar"), matrix (c (3,9,5,1, -2,8), nrow = 2), list (“green”, 12.3)) # Give names to the elements in the list. names (list_data) <- c (“1st Quarter”, “A_Matrix”, “A Inner list”) # Access the first element of the list. print (list_data [1]) # Access the thrid element. As it is also a list, all its elements will be printed. print (list_data [3]) # Access the list element using the name of the element. print (list_data $ A_Matrix)
When we run the above code, the following result is obtained:
$ `1st_Quarter` [1] “Jan” “Feb” “Mar” $ A_Inner_list $ A_Inner_list [[1]] [1] “green” $ A_Inner_list [[2]] [1] 12.3 [, 1] [, 2] [, 3] [1,] 3 5 -2 [2,] 9 1 8
Hire members from a list
We can list the elements as shown below: Add or remove. We can only add elements to the bottom of the List. Additionally, we can only delete elements from the bottom of a list. But we can Update any aspect.
# Create a list containing a vector, a matrix and a list. list_data <- list (c ("Jan", "Feb", "Mar"), matrix (c (3,9,5,1, -2,8), nrow = 2), list (“green”, 12.3)) # Give names to the elements in the list. names (list_data) <- c (“1st Quarter”, “A_Matrix”, “A Inner list”) # Add element at the end of the list. list_data [4] <- “New element” print (list_data [4]) # Remove the last element. list_data [4] <- NULL # Print the 4th Element. print (list_data [4]) # Update the 3rd Element. list_data [3] <- “updated element” print (list_data [3])
When we execute the above code, the following result is obtained:
[[1]] [1] “New element” $ <NA> NULL $ `A Inner list` [1] “updated element”
Merge Lists In R
You can merge multiple lists into a single list. This is achieved by placing all the lists within a list() function.
# Create two lists. list1 <- list (1,2,3) list2 <- list ("Sun", "Mon", "Tue") # Merge the two lists. merged.list <- c (list1, list2) # Print the merged list. print (merged.list)
When we run the above code, the following result is obtained:
[[1]] [1] 1 [[2]] [1] ۲ [[3]] [1] 3 [[4]] [1] “Sun” [[5]] [1] “Mon” [[6]] [1] “Tue”
Convert the List to a vector
A list can be converted into an array, allowing its elements to be used for future purposes. All arithmetic operations on vectors can be performed after the List has been converted to vectors.
To perform this conversion, we use the unlist() function. This function takes the List as input and generates a vector.
# Create lists. list1 <- list (1: 5) print (list1) list2 <-list (10:14) print (list2) # Convert the lists to vectors. v1 <- unlist (list1) v2 <- unlist (list2) print (v1) print (v2) # Now add the vectors result <- v1 + v2 print (result)
When we run the above code, the following result is obtained:
[[1]] [1] 1 2 3 4 5 [[1]] [1] 10 11 12 13 14 [1] 1 2 3 4 5 [1] 10 11 12 13 14 [1] 11 13 15 17 19