Learn Hello World In Java
Hello World! It is a simple program that outputs Hello, World! Prints on the screen. Because the program is so simple, it is usually used to introduce a new programming language to a new user.
Let’s check out how “Hello World!” works.
To run this program on your System, ensure Java is installed correctly. You also need an IDE (or text editor) to write and edit Java code.
Hello World application in Java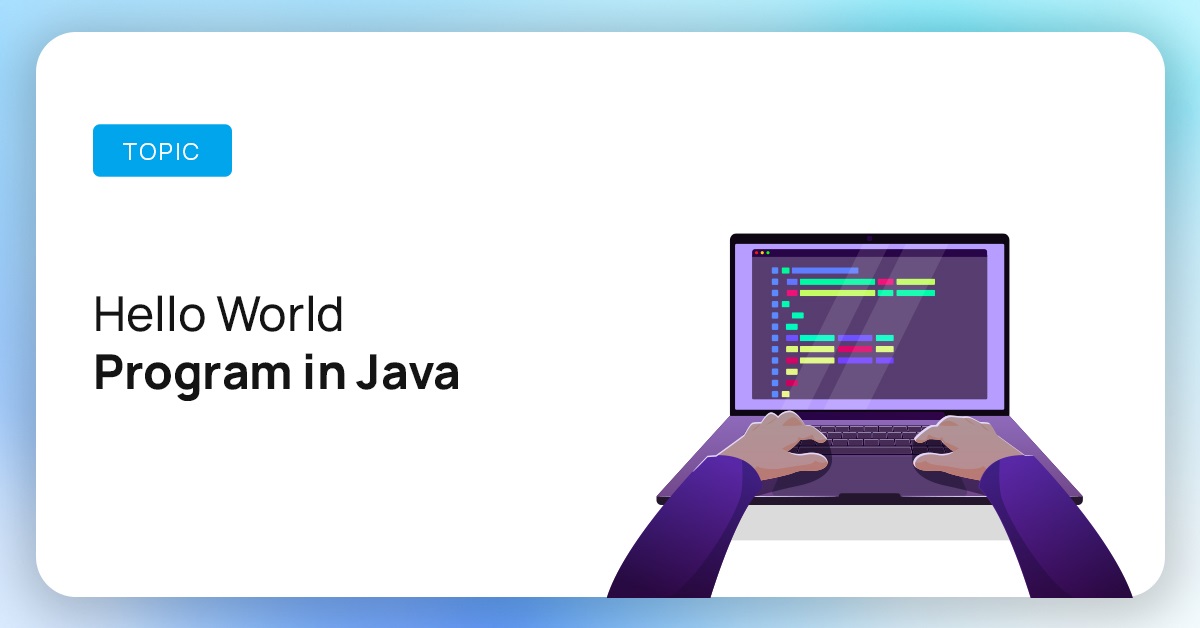
- // Your First Program
- class HelloWorld {
- public static void main (String [] args) {
- System.out.println (“Hello, World!”);
- }
- }
If you copied the code accurately, save the File name HelloWorld.java because the class name and filename in Java must match.
When you run the program, the output is:
Hello, World!
“Hello World!” How does it work in Java?
// Your First Program
In Java, any line starting with // is a comment. Comments to read the description of the code and understand more about it. The Java compiler completely ignores these lines (the application that executes Java code for the computer).
class HelloWorld……
In Java, every program starts with a class definition. In the above program, HelloWorld is the class name, and the class definition is:
class HelloWorld { … ..… }
Remember that every Java program has a class definition, and the class name must match the File name in Java.
public static void main (String [] args) {……
The top line is the primary method. Every Java program must have a main method. The Java compiler starts executing the code using the primary process.
Remember that the primary function is the Java entry point of the mandatory program. The signature of the primary function in Java is equal to:
public static void main (String [] args) {
… ..…
}
System.out.println ("Hello, World!");
The above code prints the String inside the quotation mark in the standard output (screen). Note, this is the line of code inside the primary function inside the class definition.
Important Points
- Every valid Java program must have a class definition (which matches the File name).
- The primary function must be in the class definition.
- The compiler starts executing the code from the primary function.
The following code is a valid Java program that does nothing.
public class HelloWorld { public static void main (String [] args) { // Write your code here } }
If you do not understand the meaning of class, static, methods, and so on, do not worry. We will explain them in full in the following sections.