Python – Uses and Important Features
Introduction
Python is a versatile, high-level programming language known for its simplicity, readability, and extensive ecosystem. Its wide range of applications—from web development to artificial intelligence—and its beginner-friendly design make it one of the most popular languages in 2025. This guide explores Python’s primary uses across various platforms, highlighting key features driving its adoption.
1. Uses of Python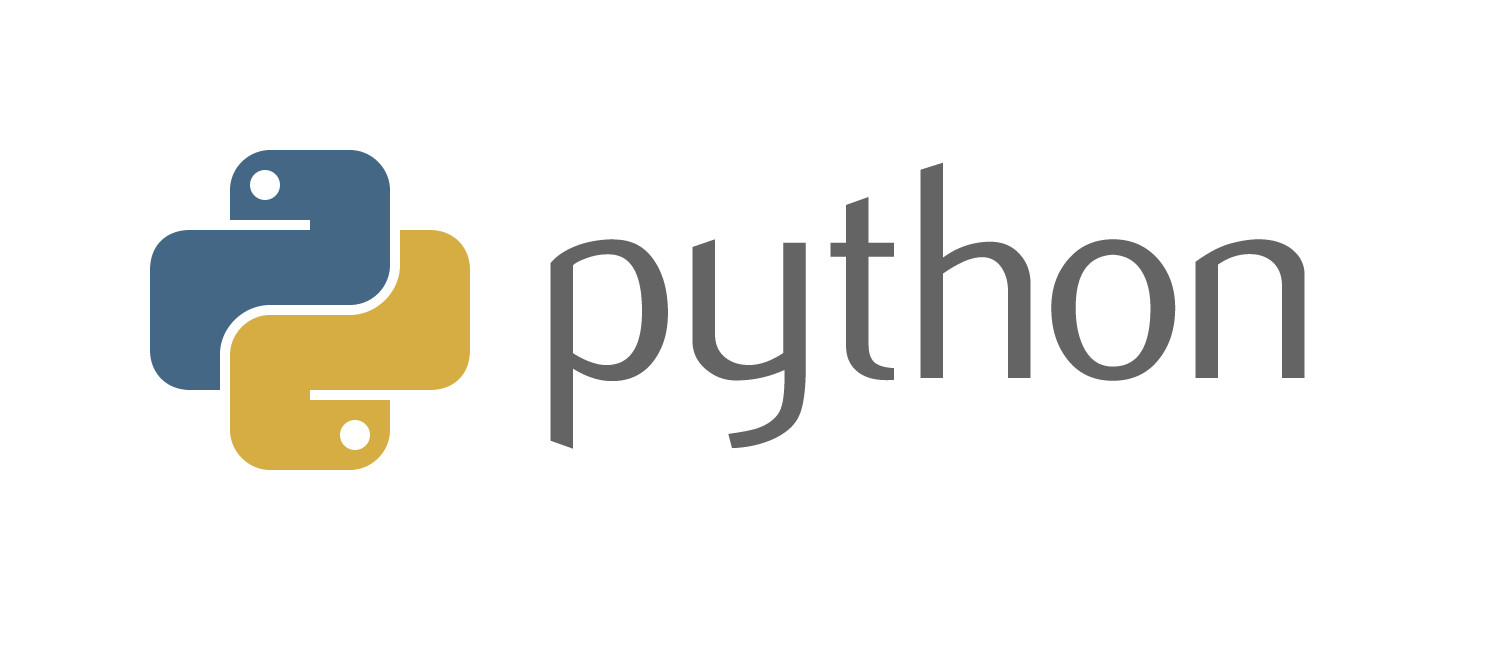
Python’s flexibility makes it suitable for various applications. Below are its major use cases, with examples to illustrate its real-world impact.
Web Development
Python powers dynamic, scalable web applications through frameworks like Django, Flask, and FastAPI.
- Applications: Building websites, REST APIs, and microservices.
- Examples: Instagram (Django), Netflix (Flask for parts of its infrastructure), and API-driven startups (FastAPI).
- Why Python? Frameworks simplify routing, database integration, and security, enabling rapid development.
Data Science and Analytics
Python is the go-to language for data analysis, visualization, and reporting.
- Applications: Data cleaning, exploratory analysis, and statistical modeling.
- Examples: Financial analysts use Pandas to process market data; companies like Spotify leverage Python for user behavior analytics.
- Why Python? Libraries like Pandas, NumPy, and Matplotlib provide intuitive tools for handling large datasets.
Machine Learning and Artificial Intelligence
Python dominates AI and machine learning (ML) due to its robust libraries and community.
- Applications: Image recognition, natural language processing (NLP), predictive modeling.
- Examples: Google’s TensorFlow powers an AI service. Google’s Face Transformers enable chatbots inspired by Grok.
- Why Python?: TensorFlow, PyTorch, and scikit-learn simplify building and deploying complex models.
Automation and Scripting
Python automates repetitive tasks, saving time and reducing errors.
- Applications: File manipulation, web scraping, system administration.
- Examples: Automating Excel reports with Pandas, scraping websites with BeautifulSoup, or scripting DevOps tasks with Python.
- Why Python?Simple syntax and libraries like
os
andshutil
Make automation accessible.
Network Security
Python enhances cybersecurity through tools for scanning, encryption, and monitoring.
- Applications: Vulnerability scanning, secure communication, and intrusion detection.
- Examples: Scapy for packet analysis, Paramiko for SSH automation, and custom scripts for log analysis.
- Why Python?Libraries like
cryptography
andscapy
Enable secure, automated solutions.
Scientific Computing
Python supports research in physics, biology, and engineering.
- Applications: Simulations, data modeling, computational biology.
- Examples: NASA uses Python for data analysis; researchers model climate change with NumPy and SciPy.
- Why Python? Libraries like SciPy and SymPy provide advanced mathematical tools.
Game Development
Python is used for prototyping and building games.
- Applications: 2D/3D games, game logic scripting.
- Examples: Pygame powers indie games like “Frets on Fire”; Unity integrates Python. Pygame and arcade libraries simplify graphics and event handling.
Internet of Things (IoT)
Python controls and processes data for IoT devices.
- Applications: Home automation, sensor data processing.
- Examples: Raspberry Pi projects for smart homes; MicroPython on microcontrollers like ESP32.
- Why Python?Lightweight libraries and cross-platform support suit resource-constrained devices.
2. Important Features of Python
Python’s design and features contribute to its popularity and versatility. Below are its key characteristics, with explanations of their significance.
Easy-to-Read Syntax
- Description: Python’s syntax is clear and resembles Python’s language, using indentation for code blocks.
- Benefit: Reduces learning curve, improves code readability, and minimizes errors.
- Example:
def greet(name): return f"Hello, {name}!" print(greet("Alice")) # Output: Hello, Alice!
- Impact: Beginners can write functional code quickly, and teams maintain code easily.
Interpreted Language
- Description: Python executes code line-by-line without compilation, using an interpreter.
- Benefit: Enables rapid development and testing in interactive environments like Jupyter Notebook.
- Example: Run
python script.py
or use a REPL to test snippets instantly. - Impact: Speeds up prototyping, though execution is slower than compiled languages like C++.
Cross-Platform Compatibility
- Description: Python runs on Windows, macOS, Linux, and embedded systems.
- Benefit: Write code once, deploy anywhere, with minimal modifications.
- Example: A web app developed on Linux (e.g., Django) runs on Windows servers.
- Impact: Simplifies deployment in diverse environments, from cloud servers to IoT devices.
Extensive Standard Library
- Description: Python includes a vast standard library for tasks like file I/O, networking, and data processing.
- Benefit: Reduces dependency on external packages for everyday tasks.
- Example:
import os print(os.listdir('.')) # Lists files in current directory
- Impact: Accelerates development by providing built-in tools.
Rich Ecosystem of Libraries
- Description: Thousands of third-party libraries (e.g., Pandas, TensorFlow, Django) extend Python’s capabilities.
- Benefit: Enable Pythonized tasks without reinventing the wheel.
- Example: Use
requests
for HTTP callsnumpy
and numerical computations. - Impact: Supports diverse applications, from AI to web development.
Dynamic Typing
- Description: Variables don’t require explicit type declaration; types are inferred at runtime.
- Benefit: Simplifies coding but requires careful error handling.
- Example:
x = 10 # Integer x = "Hello" # Now a string print(x) # Output: Hello
- Impact: Speeds up development but may lead to type-related bugs if unchecked.
Object-Oriented and Functional Programming
- Description: Supports multiple paradigms, including object-oriented (classes) and functional (e.g., lambdas, map).
- Benefit: Flexibility in choosing the best approach to a problem.
- Example:
# Object-oriented class Dog: def __init__(self, name): self.name = name def bark(self): return f"{self.name} says Woof!" dog = Dog("Rex") print(dog.bark()) # Output: Rex says Woof! # Functional numbers = [1, 2, 3] squares = list(map(lambda x: x**2, numbers)) print(squares) # Output: [1, 4, 9]
- Impact: Suits diverse coding styles and project requirements.
Community and Documentation
- Description: Python has a large, active community and extensive documentation.
- Benefit: Abundant tutorials, forums, and updates ensure support and relevance.
- Example: Access official docs at python.org or community resources on X.
- Impact: Eases learning and problem-solving.
Scalability
- Description: Python scales from small scripts to large systems with frameworks and tools like Dask or cloud platforms.
- Benefit: Supports projects of varying complexity.
- Example: Dropbox uses Python for its backend, scaling to millions of users.
- Impact: Suitable for startups and enterprises alike.
3. Practical Example: Combining Uses and Features
To illustrate Python’s versatility, here’s a simple Python example combining web scraping (automation), data analysis (data science), and readable syntax:
import requests import pandas as pd # Web scraping: Fetch data from a sample API response = requests.get("https://jsonplaceholder.typicode.com/users") data = response.json() # Data analysis: Convert to DataFrame and summarize df = pd.DataFrame(data) print("User Summary:") print(df[['name', 'email']].head()) # Save to CSV df.to_csv("users.csv", index=False)
Explanation:
- Use: Automation (scraping) and data science (analysis).
- Features: Uses
requests
(library ecosystem), Pandas (data manipulation) and straightforward syntax. - Output: Displays a table of user names and emails, saves to CSV.
- Impact: Shows how Python integrates multiple tasks in a few lines.
4. Why Python Stands Out in 2025
- Versatility: Spans web, AI, security, and more, unlike domain-specific languages.
- Adoption: Used by tech giants (Google, Meta) and startups, ensuring job opportunities.
- Trends: Supports emerging fields like quantum computing (e.g., Qiskit) and edge AI (e.g., TensorFlow Lite).
- Community: Active development (e.g., Python 3.13 in 2025) keeps it cutting-edge.
5. Best Practices
- Use Virtual Environments: Isolate dependencies with
venv
orpipenv
. - Leverage Libraries: Choose appropriate libraries (e.g., Pandas for data, Flask for web).
- Write Readable Code: Follow PEP 8 guidelines for style and clarity.
- Test Thoroughly: Use
unittest
orpytest
to catch errors. - Stay Updated: Monitor Python releases and library updates via PyPI or X posts.
6. Next Steps
- Practice: Build a small project (e.g., a Flask web app, a Pandas data analyzer).
- Learn: Explore free courses (e.g., Codecademy’s Python, Coursera’s Python).
- ExpeCoursera’sy libraries like
scikit-learn
for ML orpygame
for games. - Contribute: Join open-source projects on GitHub (e.g., Pandas, Django).
- Stay Informed: Follow Python communities on X or blogs like Real Python.
7. Conclusion
Python’s uses span web development, data science, AI, automation, security, and more. It is driven by its simple syntax, extensive libraries, and cross-platform support. Its features—readability, dynamic typing, and a vast ecosystem—make it accessible to beginners and powerful for experts.
Whether you’re building a website, analyzing data, or training neural networks, Python provides the tools to succeed. Start with small scripts, explore its libraries, and leverage its community to unlock endless possibilities.