Basic Programming Syntax Training R
For ease of use, learn R programming by writing “Hello World!” Let’s start. Depending on your needs, you can write your program, execute it in the R Command, or use an R script for this purpose.
Basic Programming Syntax Training R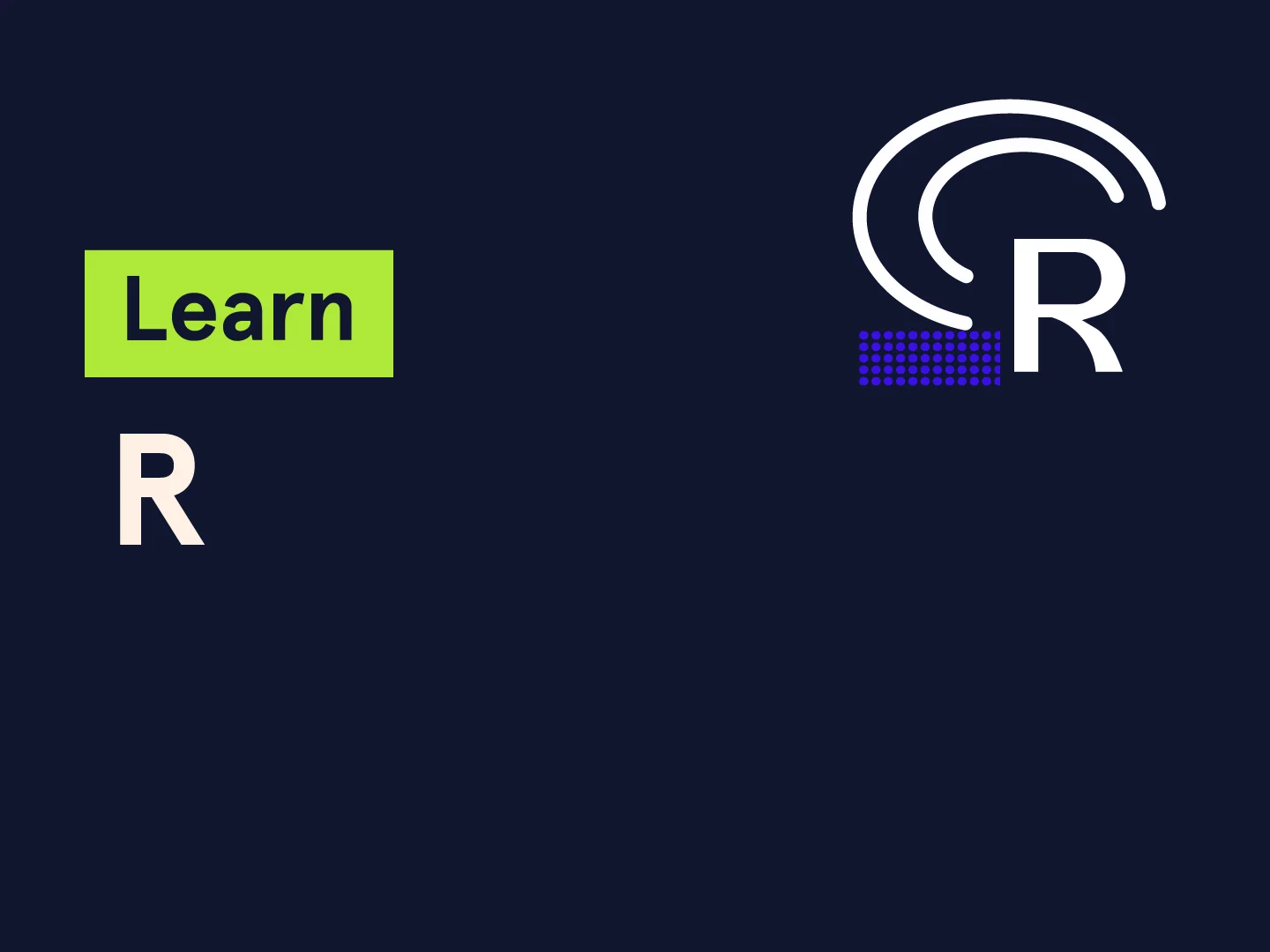
Syntax training in R: Let’s look at both.
$ R
This will launch R interpreter, and you will get to the prompt> where you can type your program as follows:
> myString <- “Hello, World!” > print (myString) [1] “Hello, World!”
Here, the first expression defines a String variable and assigns the phrase “Hello World” to it. Then, the print() statement is used to print the value stored in the myString variable.
R Script File
Typically, you will write your programs in script files and then use the R interpreter, which is invoked by Rscript, to run those scripts in the Command prompt. So, let’s proceed as follows: Start by writing the following code in the text File that tests. R calls:
# My first program in R Programming myString <- “Hello, World!” print (myString)
Save the above code to a File named test.. Run it on the Linux Command line as shown below. Even if you use Windows or other systems, the syntax you use is the same.
$ Rscript test.R
When we run the above program, the following result is obtained:
[1] "Hello, World!"
Comments
Comments are the same as the help text in your R program while it is running; the program interpreter ignores these comments. Single line comment like below: Using # at the beginning of the phrase; It is written:
# My first program in R Programming
R programming does not support multi-line comments, but you can use a trick that looks something like this:
if (FALSE) {
“This is a demo for multi-line comments, and it should be put inside either a
single OR double quote”
} myString <- “Hello, World!” print (myString) [1] “Hello, World!”
Although the R program interpreter executes the above comments, they do not interfere with your actual program. You should put these types of remarks either in one quote or in two quotes.
R – Basic Syntax
To begin learning R programming, we’ll start with a classic “Hello, World!” example. You can write and execute R code in two ways:
- Directly in the R interactive prompt
- Using an R script File
1. R Command Prompt
After setting up the R environment, launch the R interpreter by entering:
You’ll see the >
prompt, where you can type and execute R code:
> myString <- "Hello, World!" > print(myString) [1] "Hello, World!"
myString
It’s a variable storing the text"Hello, World!"
.print()
Displays the value of the variable.
2. R Script File
For more extensive programs, save your code in a script File (e.g., test.R
) and run it using Rscript:
# My first R program myString <- "Hello, World!" print(myString)
Running the Script
At the terminal (Linux/macOS/Windows), execute:
$ Rscript test.R
Output:
[1] "Hello, World!"
3. Comments in R
Comments help document code and are ignored during execution.
Single-Line Comments
Use #
:
# This is a comment
Multi-Line Comments (Workaround)
Since R doesn’t support multi-line comments directly, you can use:
if(FALSE) { "This is a pseudo multi-line comment. It’s enclosed in quotes but never executed." }
Note: The if(FALSE)
The block prevents execution, effectively acting like a comment.
This guide covers the basics of R syntax, execution methods, and commenting. Please let me know if you’d like any modifications.
Functions in R
Introduction to Functions
In R, functions are fundamental objects that (typically) take arguments and return values. These values can be any R object, including other functions. Let’s explore how functions work using examples.
Basic Function Example: sqrt()
The sqrt()
Function calculates the square root of its input:
sqrt(2) # [1] 1.414214
Vectorized Operations
R functions often work on entire vectors:
sqrt(1:10) # Applies sqrt to each element from 1 to 10 # [1] 1.000000 1.414214 1.732051 2.000000 2.236068 2.449490 2.645751 2.828427 # [9] 3.000000 3.162278
Error Handling
Functions produce errors or warnings for invalid inputs:
sqrt("hello world") # Error: non-numeric argument sqrt(-1) # Warning: NaN produced (no real square root) # [1] NaN
Function Arguments
Many functions accept multiple arguments, some of which are optional.
Example: round()
The round()
Function formats numbers to the specified decimal places:
round(pi) # Default (0 decimal places) # [1] 3 round(pi, 2) # Round to 2 decimal places (positional argument) # [1] 3.14 round(pi, digits = 2) # Same as above (named argument) # [1] 3.14
Key Points About Arguments:
- Positional Passing: Arguments can be given in order (
round(pi, 2)
). - Named Passing: Explicitly naming arguments (
digits = 2
) improves readability. - Default Values: If omitted,
digits
defaults to0
.
Exploring Function Arguments
To quickly check a function’s arguments, use args()
:
args(round) # function (x, digits = 0) # NULL
This shows that round()
requires x
(the number) and optionally accepts digits
.
Other Help Tools
?round
orhelp(round)
Detailed documentation.??round
Searches broader help topics.
Summary
- Functions process inputs and return outputs.
- Many functions are vectorized, meaning they work on entire vectors.
- Arguments can be passed positionally or by name.
- Use
args()
,?
, orhelp()
to explore functions.