Java Programming Language: History, Features, Terms, and Tips for Beginners
Java is a powerful, class-based, object-oriented programming language emphasizing minimal implementation dependencies.
It is designed to enable developers to “Write Once, Run Anywhere” (WORA), meaning compiled Java code can run on any platform supporting Java without recompilation.
Initially developed by James Gosling at Sun Microsystems in May 1995, Java was later acquired by Oracle Corporation and has since become a cornerstone for building applications across various platforms, including desktop, web, and mobile.
Java is celebrated for its simplicity, robustness, and security features, making it a popular choice for enterprise-grade applications. Java programs are compiled into bytecode, which can run on any Java Virtual Machine (JVM), ensuring platform independence.
Java’s syntax is heavily influenced by C/C++, making it familiar to developers with prior experience in these languages.
Table of Contents:
- History of Java
- Key Features of Java
- Platform Independence
- Object-Oriented Programming
- Simplicity
- Robustness
- Security
- Distributed Computing
- Multithreading
- Portability
- High Performance
- How Java Code Executes
- Program Creation
- Program Compilation
- Program Execution
- Essential Java Terminologies
- Java Virtual Machine (JVM)
- Bytecode
- Java Development Kit (JDK)
- Java Runtime Environment (JRE)
- Garbage Collector
- ClassPath
- Advantages and Disadvantages of Java
- Conclusion
History of Java: Java’s development began in 1991 when James Gosling, Mike Sheridan, and Patrick Naughton formed the “Green Team” at Sun Microsystems to create a new programming language named “Oak.”
Oak was later renamed Java, inspired by Java coffee, and officially launched in 1996 as Java 1.0. The primary objective was to provide a cross-platform runtime environment.
Arthur Van Hoff significantly refined the Java compiler to ensure strict adherence to specifications, boosting its reliability and platform independence.
Significant Milestones in Java’s Evolution:
- Java 2 introduced multiple configurations to cater to different platforms, reinforcing its versatility.
- In 1997, Sun Microsystems initiated efforts to formalize Java through ISO standards but eventually withdrew.
- On November 13, 2006, Sun Microsystems released most Java Virtual Machines as free and open-source software, further solidifying Java’s presence in the open-source community.
- By May 8, 2007, the core JVM code became fully open-source, allowing developers to innovate and extend Java’s capabilities.
Java remains a cornerstone of modern software development, widely utilized in mobile applications, enterprise systems, web services, and more.
Key Features of the Java Programming Language:
- Platform Independence: Java code is compiled into bytecode, which can be executed on any platform with a compatible JVM, making Java platform-independent.
- Object-Oriented Programming: Java is structured around objects and classes, adhering to four fundamental principles: Abstraction, Encapsulation, Inheritance, and Polymorphism.
- Simplicity: Java eliminates complex features like pointers and multiple inheritance, reducing potential bugs and enhancing code maintainability.
- Robustness: Java includes features like exception handling, garbage collection, and memory allocation, ensuring greater program stability.
- Security: Java’s runtime environment prevents unauthorized access through secure memory management, bytecode verification, and sandboxing.
- Distributed Computing: Java facilitates the development of distributed systems through Remote Method Invocation (RMI) and Enterprise JavaBeans (EJB).
- Multithreading: Java supports concurrent execution of multiple threads, improving performance in applications requiring parallel processing.
- Portability: Java bytecode can run on any platform with a JVM, promoting the “Write Once, Run Anywhere” principle.
- High Performance: Java employs Just-In-Time (JIT) compilation to optimize runtime performance by compiling frequently executed bytecode into native machine code.
Execution of Java Code:
- Creating the Program: Java source code is written using text editors or Integrated Development Environments (IDEs) like Eclipse, IntelliJ IDEA, or NetBeans and saved with a .java extension.
- Compiling the Program: The Java compiler (javac) translates the source code into bytecode, which is platform-independent and stored in .class files.
- Running the Program: The JVM executes the compiled bytecode by converting it to native machine code specific to the operating system.
Example Program:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
Essential Java Terminologies:
- Java Virtual Machine (JVM): Executes Java bytecode and ensures platform independence.
- Bytecode: Platform-independent intermediate code generated by the Java compiler.
- Java Development Kit (JDK): Includes the compiler, JVM, and libraries necessary for developing Java applications.
- Java Runtime Environment (JRE): Provides the essential libraries and JVM to run Java applications.
- Garbage Collector: Manages memory allocation and deallocation, reclaiming memory occupied by unused objects.
- ClassPath: The path where the JVM and compiler search for .class files to load external libraries.
Advantages and Disadvantages of Java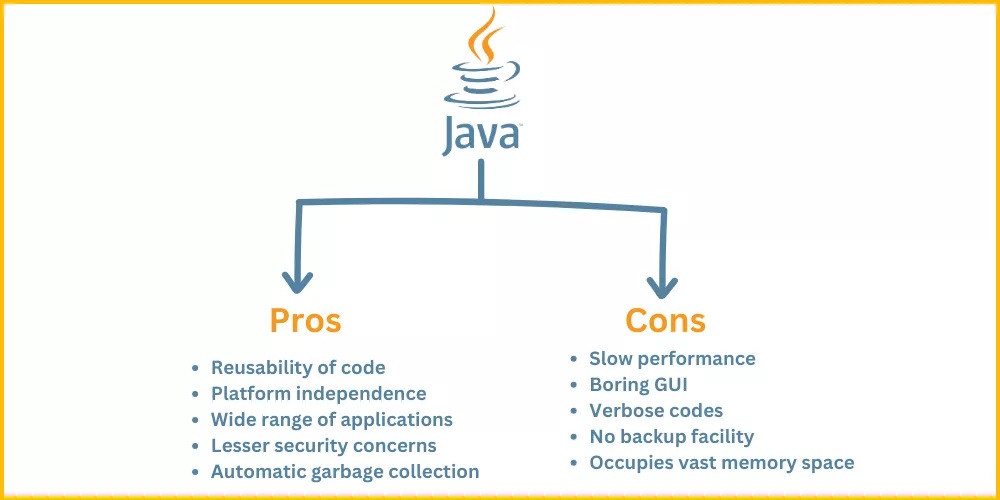
Advantages:
- Platform Independent
- Object-Oriented
- Security Features
- Large Community Support
- Enterprise-Level Applications
Disadvantages:
- Performance Overhead
- Memory Management Challenges
📌 Current Java SE Versions
Version | Release Date | LTS Status |
---|---|---|
Java SE 24 | March 18, 2025 | No |
Java SE 23 | September 17, 2024 | No |
Java SE 22 | March 19, 2024 | No |
Java SE 21 | September 19, 2023 | ✅ LTS |
Java SE 20 | March 21, 2023 | No |
Java SE 19 | September 20, 2022 | No |
Java SE 18 | March 22, 2022 | No |
Java SE 17 | September 14, 2021 | ✅ LTS |
Java SE 16 | March 16, 2021 | No |
Java SE 15 | September 15, 2020 | No |
Java SE 14 | March 17, 2020 | No |
Java SE 13 | September 17, 2019 | No |
Java SE 12 | March 19, 2019 | No |
Java SE 11 | September 25, 2018 | ✅ LTS |
Java SE 10 | March 20, 2018 | No |
Java SE 9 | September 21, 2017 | No |
Java SE 8 | March 18, 2014 | ✅ LTS |
Note: Java SE 25 is scheduled for release in September 2025 and is planned to be the next LTS version.
Conclusion:
Java’s strength lies in its cross-platform capabilities, robust security features, and object-oriented architecture. With a strong community and extensive libraries, Java remains a preferred language for building complex enterprise applications, mobile apps, and web-based systems.
As technology evolves, Java adapts, maintaining its relevance in modern software development.