Database Models in Python and Django
In Django, database models are Python classes that define the structure of database tables. They use the ORM (Object-Relational Mapping) to map class attributes to table columns.
They simplify CRUD operations and relationships (e.g., ForeignKey, ManyToMany) by abstracting SQL, enabling intuitive database interactions.
Introduction
Django is a high-level Python web framework that simplifies building robust, scalable applications. A core feature is its Object-Relational Mapping (ORM) system.
It allows developers to define database models as Python classes and interact with databases using Python code instead of raw SQL. This makes database operations intuitive and secure.
This guide provides a comprehensive overview of database models in Django, covering model creation, relationships, migrations, and querying.
You’ll learn to design and manage databases effectively through practical examples (e.g., a blog application). By the end, you’ll be equipped to build data-driven applications with Django’s ORM.
1. Understanding Django’s ORM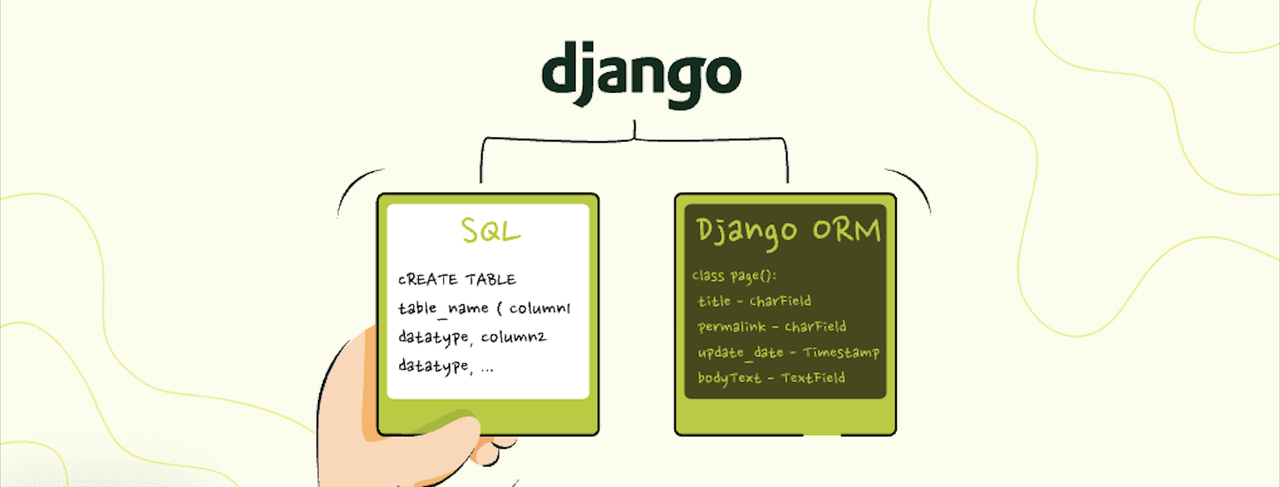
What is a Database Model?
A database model in Django is a Python class representing a database table. Each class instance corresponds to a row in the table, and class attributes define table columns (fields).
Analogy: Think of a model as a blueprint for a table. The blueprint specifies column names and types (e.g., text, integer); each object created from it is a record in the table.
What is the ORM?
Django’s ORM maps Python classes to database tables, abstracting SQL operations. You write Python code to create, read, update, and delete (CRUD) data, and Django translates it into SQL for databases like PostgreSQL, MySQL, or SQLite.
Benefits:
- Abstraction: No need to write SQL queries.
- Portability: Works with multiple databases (e.g., switch from SQLite to PostgreSQL).
- Security: Prevents SQL injection by sanitizing inputs.
- Productivity: Simplifies complex operations like joins and filtering.
2. Setting Up Django
To follow the examples, set up a Django project:
- Install Django:
pip install django
- Create a project:
django-admin startproject blog_project
- Create an app:
cd blog_project && python manage.py startapp blog
- Add the app to
INSTALLED_APPS
inblog_project/settings.py
:INSTALLED_APPS = [ ... 'blog.apps.BlogConfig', ] Configure the database in blog_project/settings.py (SQLite is default): DATABASES = { 'default': { 'ENGINE': 'django.db.backends.sqlite3', 'NAME': BASE_DIR / 'db.sqlite3', } }
3. Creating Database Models
Models are defined in an app’s models.py
file. Each model inherits from django.db.models.Model
.
Example: Blog Application Models
Let’s create models for a blog with posts and categories.
# blog/models.py from django.db import models class Category(models.Model): name = models.CharField(max_length=100, unique=True) description = models.TextField(blank=True) def __str__(self): return self.name class Meta: verbose_name_plural = "categories" class Post(models.Model): title = models.CharField(max_length=200) content = models.TextField() created_at = models.DateTimeField(auto_now_add=True) updated_at = models.DateTimeField(auto_now=True) category = models.ForeignKey(Category, on_delete=models.CASCADE, related_name='posts') def __str__(self): return self.title
Explanation:
- Fields:
CharField
: Fixed-length string (e.g.,name
,title
).TextField
Unlimited text (e.g.,description
,content
).DateTimeField
Date and time, withauto_now_add
(set on creation) andauto_now
(set on update).ForeignKey
Defines a one-to-many relationship (e.g., one category has many posts).
on_delete=models.CASCADE
Deletes posts if their category is deleted.related_name='posts'
: Allows reverse queries (e.g.,category.posts.all()
).__str__
Returns a human-readable representation of the model.Meta
Customizes model behavior (e.g., plural name for admin interface).
4. Model Relationships
Django supports three main types of relationships:
- One-to-Many (ForeignKey): One record relates to multiple records (e.g., one category, many posts).
- Many-to-Many (ManyToManyField): Multiple records relate to various records (e.g., posts with multiple tags).
- One-to-One (OneToOneField): One record relates to exactly one record (e.g., a user profile).
Example: Adding Many-to-Many for Tags
# blog/models.py (updated) class Tag(models.Model): name = models.CharField(max_length=50, unique=True) def __str__(self): return self.name class Post(models.Model): title = models.CharField(max_length=200) content = models.TextField() created_at = models.DateTimeField(auto_now_add=True) updated_at = models.DateTimeField(auto_now=True) category = models.ForeignKey(Category, on_delete=models.CASCADE, related_name='posts') tags = models.ManyToManyField(Tag, related_name='posts', blank=True) def __str__(self): return self.title
Explanation:
ManyToManyField
Links posts to multiple tags and tags to various posts.blank=True
Allows posts to have no tags.- Reverse Queries: Access posts via
tag.posts.all()
.
5. Migrations
Django uses migrations to apply model changes to the database schema.
Steps
- Create Migrations: After defining models, generate migration files.
python manage.py makemigrations
This creates files
blog/migrations/
describing schema changes. - Apply Migrations: Update the database.
python manage.py migrate
This creates tables (e.g.,
blog_category
,blog_post
,blog_tag
).
Example Output:
Operations to perform: Apply all migrations: admin, auth, blog, contenttypes, sessions Running migrations: Applying blog.0001_initial... OK
Note: If you modify models (e.g., add a field), rerun makemigrations
and migrate
.
6. Querying the Database
Django’s ORM provides a powerful query API for CRUD operations.
Example: CRUD Operations
Set up a Django shell to test queries:
python manage.py shell # Import models from blog.models import Category, Post, Tag from django.utils import timezone # Create data category = Category.objects.create(name="Tech", description="Technology topics") post = Post.objects.create( title="Django Basics", content="Learn Django ORM", category=category ) tag = Tag.objects.create(name="Python") post.tags.add(tag) # Read data all_posts = Post.objects.all() # Get all posts tech_posts = Post.objects.filter(category__name="Tech") # Filter by category post_detail = Post.objects.get(title="Django Basics") # Get single post # Update data post.content = "Updated content" post.save() # Delete data post.delete() # Print results print(f"All Posts: {list(all_posts)}") print(f"Tech Posts: {list(tech_posts)}") print(f"Post Tags: {list(post_detail.tags.all())}")
Explanation:
- Create: Use
create()
orsave()
to add records. - Read:
all()
Retrieves all records.filter()
Returns a queryset matching conditions.get()
: Retrieves a single record (raises an error if none/multiple are found).
- Update: Modify attributes and call
save()
. - Delete: Call
delete()
to remove records. - Relationships: Access related objects (e.g.,
post.tags.all()
,category.posts.all()
).
Sample Output:
All Posts: [<Post: Django Basics>] Tech Posts: [<Post: Django Basics>] Post Tags: [<Tag: Python>]
Advanced Queries
- Chaining Filters:
recent_tech_posts = Post.objects.filter(category__name="Tech", created_at__gte=timezone.now().date())
- Aggregations:
from django.db.models import Count category_counts = Category.objects.annotate(post_count=Count('posts')) for cat in category_counts: print(f"{cat.name}: {cat.post_count} posts")
- Q Objects for Complex Queries:
from django.db.models import Q posts = Post.objects.filter(Q(title__icontains="Django") | Q(content__icontains="ORM"))
7. Admin Interface Integration
Django’s admin interface automatically generates a UI for managing models.
Steps
- Register models in
blog/admin.py
:# blog/admin.py from django.contrib import admin from .models import Category, Post, Tag admin.site.register(Category) admin.site.register(Post) admin.site.register(Tag)
- Create a superuser:
python manage.py createsuperuser
- Run the server:
python manage.py runserver
- Access
http://127.0.0.1:8000/admin/
and log in to manage data.
Customization (Optional):
# blog/admin.py @admin.register(Post) class PostAdmin(admin.ModelAdmin): list_display = ('title', 'category', 'created_at') list_filter = ('category', 'tags') search_fields = ('title', 'content')
Explanation:
list_display
Shows columns in the admin list view.list_filter
Adds filters for categories and tags.search_fields
Enables searching by title and content.
8. Best Practices
- Model Design:
- Use meaningful field names (e.g.,
title
overt
). - Set appropriate constraints (e.g.,
unique=True
,blank=False
). - Define
__str__
for readable object representations.
- Use meaningful field names (e.g.,
- Relationships:
- Use
related_name
for clarity in reverse queries. - Choose the right relationship type (e.g.,
ForeignKey
vs.ManyToManyField
).
- Use
- Migrations:
- Test migrations in development before production.
- Backup databases before applying migrations.
- Query Optimization:
- Use
select_related()
For ForeignKey joins:Post.objects.select_related('category')
. - Use
prefetch_related()
For ManyToMany/reverse joins:Post.objects.prefetch_related('tags')
. - Avoid excessive queries (N+1 problem) with tools like
django-debug-toolbar
.
- Use
- Security:
- Leverage Django’s ORM to prevent SQL injection.
- Restrict admin access with strong passwords and permissions.
9. Modern Trends (2025)
- Async ORM: Django 5.x (2025) supports async queries (
async for
,await
), improving performance for I/O-bound tasks. - Cloud Integration: Models integrate with cloud databases (e.g., AWS RDS, Google Cloud SQL) via Django’s database backends.
- Schema Evolution: Tools like
django-evolve
simplify complex migrations. - GraphQL Support: Libraries like
graphene-django
enhance model querying for modern APIs.
10. Next Steps
- Practice: Extend the blog app with user models, comments, or views/templates.
- Learn: Explore Django’s official docs (djangoproject.com) or courses (e.g., Codecademy’s Django, Real Python).
- Experiment: Try PostgreSQL instead of SQLite or add a REST API with Django REST Framework.
- Contribute: Join open-source Django projects on GitHub.
- Stay Updated: Follow Django’s blog or X posts from Django contributors.
11. Conclusion
Django’s ORM and database models simplify building data-driven applications by abstracting SQL into Python classes. With models, you define tables, relationships, and constraints, while the ORM handles querying and migrations.
The blog example demonstrates creating models, managing relationships, and performing CRUD operations, providing a foundation for real-world projects.
Start with the provided code, experiment with your models, and leverage Django’s ecosystem to build robust applications.