Python libraries and Their Applications
Python libraries are a collection of pre-written code (modules and packages) that provide reusable functionalities. Instead of writing code from scratch for everyday tasks, you can import and utilize these libraries to streamline your development process.
Python Libraries and Their Applications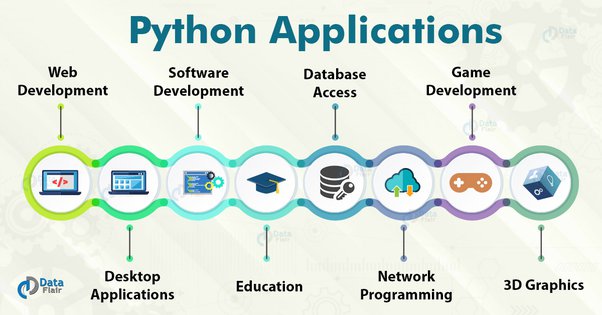
- Modules: A single Python file (
.py
extension) containing Python definitions and statements. - Packages: A collection of related modules organized in a directory hierarchy, with each directory containing a special
__init__.py
file (which can be empty or contain initialization code). - Libraries: Often used as an umbrella term to refer to modules and packages that offer a set of functionalities for a specific purpose.
The Python Standard Library (Batteries Included)
Python boasts a rich standard library that comes bundled with the interpreter. This “batteries included” philosophy means you can access many tools without installing anything extra. Here are some key modules and their applications:
1. Core Utilities:
osIt provides
functions for interacting with the operating system (e.g., file and directory manipulation, environment variables, and process management).Code snippet
import os # Get the current working directory cwd = os.getcwd() print(f"Current working directory: {cwd}") # List files and directories in the current directory items = os.listdir() print(f"Items in current directory: {items}") # Create a new directory # os.makedirs("new_directory", exist_ok=True) # Check if a file exists file_exists = os.path.exists("my_file.txt") print(f"my_file.txt exists: {file_exists}")
sys
This provides access to system-specific parameters and functions (e.g., command-line arguments, exit status, and standard input/output/error streams).Python
import sys # Get command-line arguments args = sys.argv print(f"Command-line arguments: {args}") # Exit the program with a specific status code # sys.exit(0)
datetime
Enables working with dates and times (e.g., creating, manipulating, and formatting dates and times).Python
import datetime now = datetime.datetime.now() print(f"Current date and time: {now}") today = datetime.date.today() print(f"Today's date: {today}") delta = datetime.timedelta(days=7) future_date = today + delta print(f"Date in 7 days: {future_date}")
time
Provides time-related functions (e.g., pausing execution, getting system time).Python
import time print("Start") time.sleep(2) # Pause for 2 seconds print("End") current_time = time.time() print(f"Current timestamp (seconds since epoch): {current_time}")
math
: Offers mathematical functions (e.g., trigonometric, logarithmic, exponential).Python
import math square_root = math.sqrt(16) print(f"Square root of 16: {square_root}") sin_value = math.sin(math.pi / 2) print(f"Sine of pi/2: {sin_value}")
random
Generates pseudo-random numbers for various distributions.Python
import random random_integer = random.randint(1, 10) print(f"Random integer between 1 and 10: {random_integer}") random_float = random.random() # Between 0.0 and 1.0 print(f"Random float: {random_float}") my_list = [1, 2, 3, 4, 5] random.shuffle(my_list) print(f"Shuffled list: {my_list}")
2. File and Data Handling:
ioIt provides
Tools for working with various I/O streams (e.g., in-memory and file streams).json
: Enables encoding and decoding JSON (JavaScript Object Notation) data.Python
import json data = {"name": "Alice", "age": 30, "city": "New York"} json_string = json.dumps(data) print(f"JSON string: {json_string}") parsed_data = json.loads(json_string) print(f"Parsed data: {parsed_data['name']}, {parsed_data['age']}")
csv
Facilitates working with CSV (Comma Separated Values) files.Python
import csv # Reading CSV with open('data.csv', 'r') as file: reader = csv.reader(file) for row in reader: print(row) # Writing CSV with open('output.csv', 'w', newline='') as file: writer = csv.writer(file) writer.writerow(["Name", "Age", "City"]) writer.writerow(["Bob", "25", "London"])
pickle
Allows serialization and deserialization of Python objects (converting objects to a byte stream and vice versa). Caution: Only unpickle data from trusted sources, as it can be a security risk.Python
import pickle my_dict = {"a": 1, "b": 2} serialized_data = pickle.dumps(my_dict) deserialized_data = pickle.loads(serialized_data) print(f"Deserialized data: {deserialized_data}")
3. Networking and Internet:
socket
Provides a low-level networking interface for creating network connections.http.client
andurllib
: Modules for making HTTP requests and working with URLs.Python
import urllib.request try: with urllib.request.urlopen('https://www.example.com') as response: html = response.read().decode('utf-8') # print(html[:100]) # Print the first 100 characters except urllib.error.URLError as e: print(f"Error fetching URL: {e}")
smtplib
: Enables sending emails using the Simple Mail Transfer Protocol (SMTP).ftplib
Provides functions for interacting with FTP (File Transfer Protocol) servers.
4. Concurrency and Parallelism:
threading
Supports creating and managing threads for concurrent execution.multiprocessing
Enables creating and managing processes for parallel execution (utilizing multiple CPU cores).asyncioIt provides
Infrastructure for writing concurrent code using asynchronous I/O, which is often used for network-bound and I/O-bound operations.
5. Data Compression and Archiving:
zlib
,gzip
,bz2
,lzma
: Modules for working with various compression formats.tarfile
,zipfile
: Modules for creating and extracting tar and zip archives.
6. Other Useful Modules:
re
Provides regular expression operations for pattern matching in strings.collections
: Offers specialized container datatypes beyond the built-inlist
,tuple
,dict
, andset
(e.g.,Counter
,defaultdict
,OrderedDict
).itertools
Provides tools for creating efficient iterators for looping.functools
: Offers higher-order functions and operations on callable objects (e.g.,partial
,lru_cache
).unittest
Python’s built-in framework for writing and running unit tests.logging
Provides a flexible system for emitting event messages for applications.argparse
: Helps in parsing command-line arguments.sqlite3
Enables working with SQLite databases.
Third-Party Libraries: Expanding Python’s Capabilities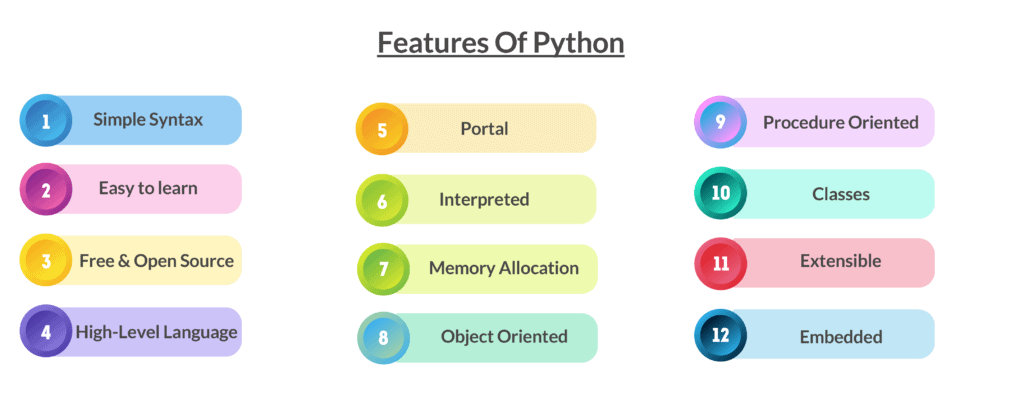
Beyond the standard library, a vast ecosystem of third-party libraries, managed primarily through the Python Package Index (PyPI), extends Python’s capabilities to virtually any domain. You typically install these using. pip
(Python Package Installer).
Here are some prominent categories and popular libraries within them:
1. Data Science and Machine Learning:
NumPy
The fundamental package for numerical computation in Python. Provides support for large, multi-dimensional arrays and matrices, along with a collection of high-level mathematical functions to operate on these arrays.Python
import numpy as np a = np.array([1, 2, 3]) b = np.array([[4, 5], [6, 7]]) print(a) print(b) print(np.dot(a, [1, 1, 1])) # Dot product
Pandas
Provides data structures for data analysis, primarily theDataFrame
(a tabular data structure) andSeries
(a one-dimensional labeled array). Offers powerful tools for data manipulation, cleaning, analysis, and visualization.Python
import pandas as pd data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [30, 25, 35], 'City': ['NY', 'London', 'Paris']} df = pd.DataFrame(data) print(df) print(df['Age'].mean())
Matplotlib
A comprehensive library for creating static, interactive, and animated visualizations in Python.Python
import matplotlib.pyplot as plt x = np.linspace(0, 10, 100) y = np.sin(x) plt.plot(x, y) plt.xlabel("X-axis") plt.ylabel("Y-axis") plt.title("Sine Wave") plt.show()
Seaborn
A data visualization library built on Matplotlib provides a higher-level interface for creating informative and attractive statistical graphics.Scikit-learn
A powerful library for machine learning tasks, including classification, regression, clustering, dimensionality reduction, model selection, and preprocessing.Python
from sklearn.linear_model import LinearRegression import numpy as np X = np.array([[1], [2], [3], [4]]) y = np.array([2, 4, 5, 4]) model = LinearRegression() model.fit(X, y) print(model.predict([[5]]))
TensorFlow
andPyTorch
Deep learning frameworks are widely used for building and training neural networks. They offer extensive tools for numerical computation, automatic differentiation, and GPU acceleration.
2. Web Development:
Flask
A lightweight and flexible micro web framework for building web applications with Python.Django
A high-level Python web framework that follows the “batteries included” approach, providing many built-in features for rapid development of complex web applications.Requests
A popular library for making human-friendly HTTP requests.Python
import requests response = requests.get('https://api.github.com/events') if response.status_code == 200: data = response.json() # print(data[0]) else: print(f"Request failed with status code: {response.status_code}")
Beautiful Soup
A library for parsing HTML and XML documents, making it easy to scrape data from websites.Selenium
A tool for automating web browsers, often used for scraping and testing web applications.
3. GUI (Graphical User Interface) Development:
Tkinter
Python’s standard GUI library provides basic widgets for creating desktop applications.PyQt
andPySide
Powerful cross-platform GUI toolkits based on the Qt framework.Kivy
An open-source Python framework for developing multi-touch applications with a natural user interface.
4. Scientific Computing and Engineering:
SciPy
A library that builds on NumPy and provides a collection of numerical algorithms and tools for scientific and technical computing (e.g., optimization, integration, interpolation, signal processing, statistics).SymPy
A library for symbolic mathematics, allowing you to perform algebraic calculations, calculus, and more symbolically.OpenCV
A comprehensive library for computer vision tasks.NLTK
(Natural Language Toolkit)A leading platform for working with human language data.SpaCy
Another popular library for advanced Natural Language Processing.
5. Game Development:
Pygame
A set of Python modules designed for writing video games.Pyglet
A cross-platform windowing and multimedia library for Python, suitable for games and other visual applications.
6. Other Domains:
SQLAlchemy
A powerful SQL toolkit and Object-Relational Mapper (ORM) for working with databases.Pillow
(PIL Fork)A library for image processing.Cryptography
Provides cryptographic primitives and recipes.pytest
andnose2
Alternative testing frameworks.Celery
A distributed task queue for asynchronous task processing.
Installing and Managing Libraries with pip
pip
is the package installer for Python. It allows you to easily install, upgrade, and uninstall third-party libraries from PyPI.
Common pip
commands:
pip install <library_name>
Installs the specified library.Bash
pip install requests
pip install <library_name>==<version>
Installs a specific version of the library.Bash
pip install pandas==1.2.0
pip uninstall <library_name>
Uninstalls the specified library.Bash
pip uninstall requests
pip show <library_name>
Displays information about an installed library.Bash
pip show pandas
pip list
Lists all installed packages.pip freeze > requirements.txt
: Creates a Arequirements.txt
file listing all installed packages and their versions, helpful in replicating environments.pip install -r requirements.txt
Installs all packages listed in therequirements.txt
file.pip upgrade <library_name>
Upgrades the specified library to the latest version.Bash
pip upgrade pandas
Conclusion
Python’s extensive standard library and the vast ecosystem of third-party libraries make it a versatile and powerful language. Whether you’re interested in data science, web development, game creation, or system administration, there’s likely a Python library that can significantly simplify your work.
By understanding the capabilities of these libraries and how to manage them with pip
You unlock the full potential of Python for your projects.