Phrases, Commands, And Blocks in Java
This tutorial teaches you about expressions, statements, blocks, and the difference between a phrase and a Command. We used phrases, commands, and blocks in previous tutorials without discussing them in detail.
Now that you know what variables, operators, and literals are, it will be easier to understand these concepts.
Java Expressions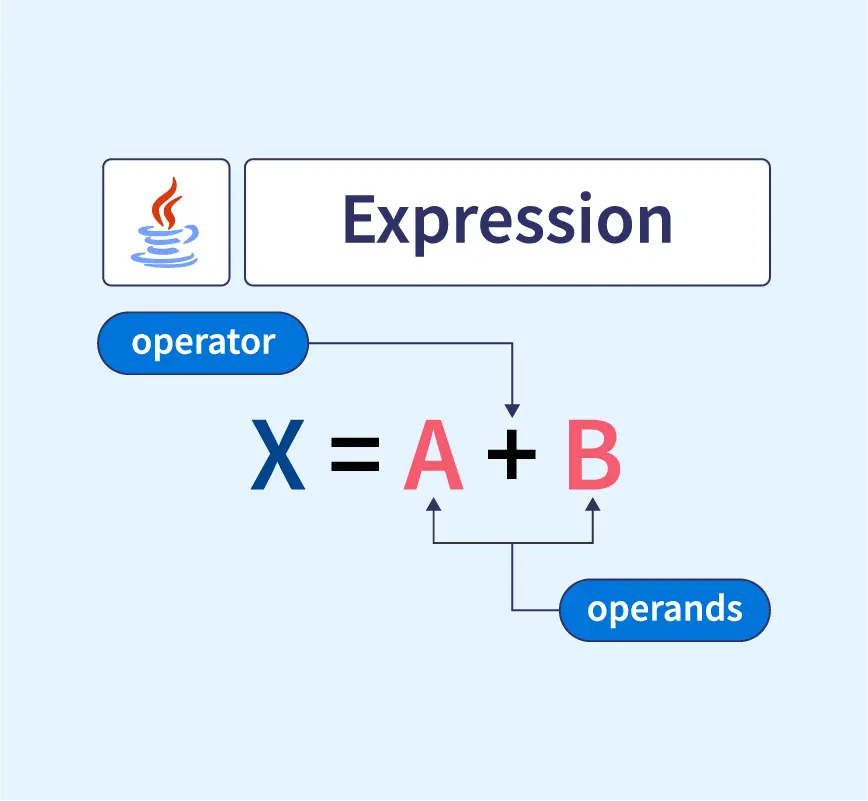
Expressions include variables, operators, literals, and method calls that evaluate a single value.
Let’s give an example:
int score; score = 90; Here, score = 90 is the expression that returns int. Double a = 2.2, b = 3.4, result; result = a + b - 3.4; Here, a + b is 3.4. if (number1 == number2) System.out.println (“Number 1 is larger than number 2”);
Here, number2 == number1 is the expression that Boolean returns. Similarly, “Number 1 is larger than number 2” is a String expression.
Java Statements
Commands form a complete execution unit. For example,
int score = 9 * 5;
Here, 9 * 5 is a statement that returns 45, and int score = 9 * 5 is a Command.
Phrases are part of commands.
Grammatical expressions
Some expressions can be used with; Terminated are known as commands. For example:
number = 10;
Here, number = 10 is a phrase, and number = 10 is a command that the compiler can execute.
++ number;
Here, ++ number is the expression while ++ number; Is a Command.
Definitive commands
Defines commands that define variables. For example,
Double tax = 9.5;
The above Command defines the tax variable with an initial value of 9.5.
Control flow commands are also used in decision making and loops in Java. You will learn control flow commands in later tutorials.
3- Java block
A block is a group of expressions (zero or more) enclosed in parentheses. For example,
- class AssignmentOperator {
- public static void main (String [] args) {
- String band = “Beatles”;
- if (band == “Beatles”) {// start of block
- System.out.print (“Hey”);
- System.out.print (“Jude!”);
- } // end of block
- }
- }
Above are two phrases
System.out.print ("Hey");
And
System.out.print (“Jude!”);
Inside the listed block.
A block may have no expression. Consider the following examples:
class AssignmentOperator { public static void main (String [] args) { if (10> 5) {// start of block } // end of block } } class AssignmentOperator { public static void main (String [] args) {// start of block } // end of block }