Understanding Classes and Object-Oriented Programming in Python
Introduction
In Python, a class is a blueprint for creating objects—individual instances that combine data (attributes) and behavior (methods). Classes are central to object-oriented programming (OOP), a programming paradigm that organizes code into reusable, modular units.
OOP makes complex programs easier to manage, scale, and maintain, powering everything from web applications to machine learning models.
This guide explains what classes are, how they relate to OOP principles, and how to use them in Python. Through examples, you’ll learn to create courses, instantiate objects, and apply OOP concepts like encapsulation, inheritance, and polymorphism.
1. What is Object-Oriented Programming?
OOP is a way of structuring code around objects, which are instances of classes. Think of a class as a template (e.g., a car blueprint) and an object as a specific instance (e.g., a red Toyota Corolla). OOP emphasizes four key principles:
- Encapsulation: Bundling data and methods together, controlling access to protect data.
- Inheritance: Allowing one class to inherit attributes and methods from another, promoting code reuse.
- Polymorphism: Allowing objects to be treated as instances of a parent class, even if they belong to a subclass, allows flexible behavior.
- Abstraction: Hiding complex details and exposing only necessary functionality.
Python’s class system supports these principles, making it a powerful tool for OOP.
2. What is a Class in Python?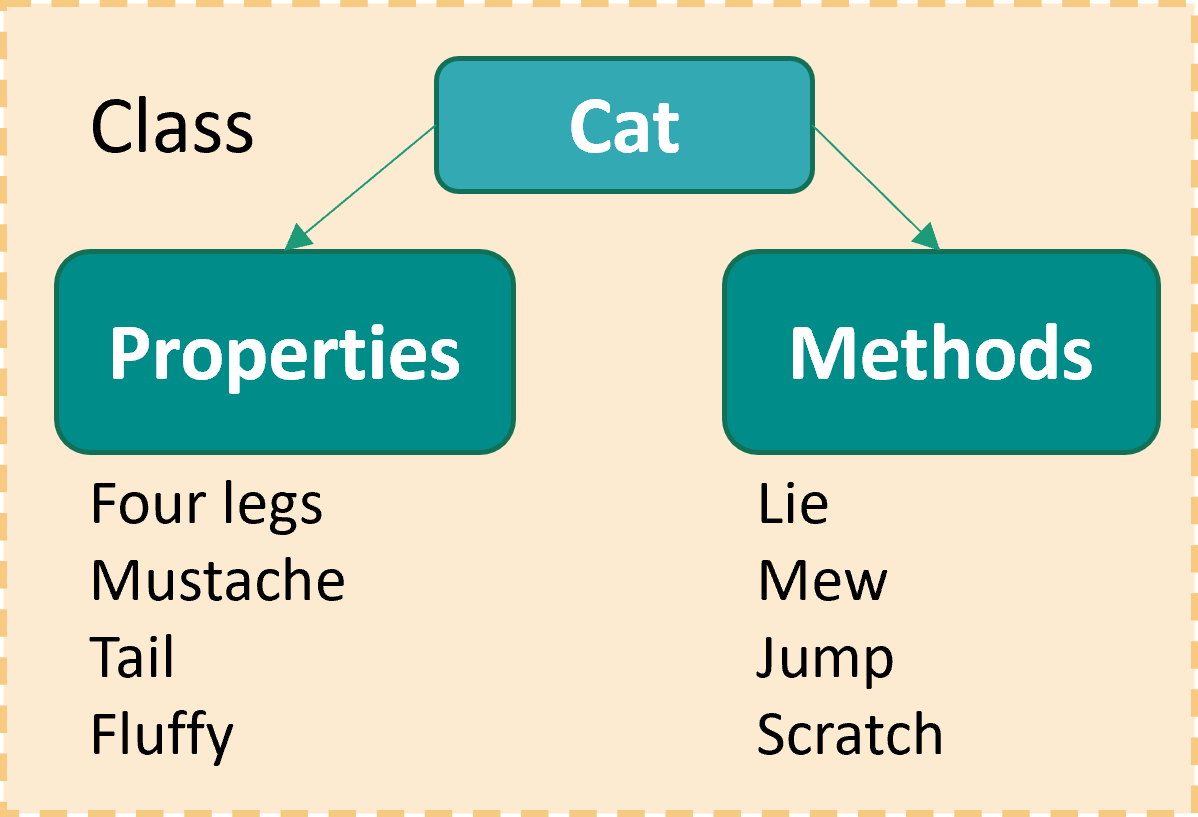
A class defines the structure and behavior of objects. It specifies:
- Attributes: Data associated with the object (e.g., a car’s color).
- Methods: Functions that define the object’s behavior (e.g., a car’s ability to drive).
Basic Syntax
Here’s a simple class definition:
class Car: def __init__(self, brand, color): self.brand = brand # Instance attribute self.color = color def drive(self): # Instance method return f"The {self.color} {self.brand} is driving!"
class
Keyword: Declares a class namedCar
.__init__
Method: The constructor initializes attributes when an object is created.self
: Refers to the instance, allowing access to its attributes and methods.- Attributes:
brand
andcolor
store data for eachCar
object. - Methods:
drive
Defines behavior.
Creating Objects
You instantiate a class to create an object:
my_car = Car("Toyota", "Red") # Create an object print(my_car.brand) # Output: Toyota print(my_car.drive()) # Output: The Red Toyota is driving!
Each object (my_car
) is an independent instance with its attributes.
3. Classes and OOP Principles
Classes in Python directly support OOP principles. Let’s explore with examples.
Encapsulation
Encapsulation bundles data and methods, often restricting access to some components to prevent unintended changes. Python uses naming conventions for access control:
- Public: Attributes/methods (e.g.,
brand
) are accessible everywhere. - Protected: Attributes with a single underscore (e.g.,
_brand
) suggest internal use (convention, not enforced). - Private: Attributes with double underscores (e.g.,
__brand
) trigger name mangling, making them harder to access outside the class.
Example:
class BankAccount: def __init__(self, owner, balance): self.owner = owner self.__balance = balance # Private attribute def deposit(self, amount): if amount > 0: self.__balance += amount return f"Deposited ${amount}. New balance: ${self.__balance}" return "Invalid deposit amount" def get_balance(self): # Public method to access private attribute return self.__balance account = BankAccount("Alice", 1000) print(account.deposit(500)) # Output: Deposited $500. New balance: $1500 print(account.get_balance()) # Output: 1500 # print(account.__balance) # Error: Attribute not directly accessible
Why It Matters: Encapsulation protects .__balance
from external modification, ensuring deposits follow the deposit
method’s logic.
Inheritance
Inheritance allows a class (child) to inherit attributes and methods from another class (parent), enabling code reuse.
Example:
class Vehicle: # Parent class def __init__(self, brand): self.brand = brand def start_engine(self): return f"{self.brand}'s engine started!" class Car(Vehicle): # Child class inherits from Vehicle def __init__(self, brand, color): super().__init__(brand) # Call parent's __init__ self.color = color def drive(self): return f"The {self.color} {self.brand} is driving!" my_car = Car("Honda", "Blue") print(my_car.start_engine()) # Output: Honda's engine started! print(my_car.drive()) # Output: The Blue Honda is driving!
super()
Calls the parent class’s methods.- Extending:
Car
inheritsstart_engine
fromVehicle
and adds its owndrive
method.
Why It Matters: Inheritance reduces duplication by sharing standard functionality across related classes.
Polymorphism
Polymorphism allows different classes to be treated as instances of a typical parent class, with each class implementing methods.
Example:
class Motorcycle(Vehicle): def start_engine(self): # Override parent's method return f"{self.brand}'s motorcycle engine roars!" vehicles = [Car("Toyota", "Red"), Motorcycle("Yamaha")] for vehicle in vehicles: print(vehicle.start_engine()) # Different outputs based on object type
Output:
Toyota's engine started!
Yamaha's motorcycle engine roars!
Why It Matters: Polymorphism enables flexible code that works with objects of different types, as long as they share a standard interface (e.g., start_engine
).
Abstraction
Abstraction hides complex implementation details, exposing only what’s necessary. Python supports this through abstract base classes (ABCs) in the abc
module.
Example:
from abc import ABC, abstractmethod class Animal(ABC): @abstractmethod def make_sound(self): pass class Dog(Animal): def make_sound(self): return "Woof!" class Cat(Animal): def make_sound(self): return "Meow!" animals = [Dog(), Cat()] for animal in animals: print(animal.make_sound()) # Output: Woof!, Meow!
ABC
and@abstractmethod
Ensure subclasses implementmake_sound
.- Cannot Instantiate
Animal
It’s abstract, enforcing a contract for subclasses.
Why It Matters: Abstraction ensures consistent interfaces, simplifying code maintenance.
4. Practical Example: Library Management System
Let’s tie everything together with a more complex example: a simple library system using classes and OOP principles.
class Book: def __init__(self, title, author, isbn): self.title = title self.author = author self.__isbn = isbn # Private self._is_checked_out = False # Protected def check_out(self): if not self._is_checked_out: self._is_checked_out = True return f"{self.title} checked out." return f"{self.title} is already checked out." def return_book(self): if self._is_checked_out: self._is_checked_out = False return f"{self.title} returned." return f"{self.title} is not checked out." class Library: def __init__(self): self.books = [] def add_book(self, book): self.books.append(book) return f"Added {book.title} to library." def find_book(self, title): for book in self.books: if book.title.lower() == title.lower(): return book return None # Usage library = Library() book1 = Book("Python Programming", "John Doe", "12345") book2 = Book("Data Science", "Jane Smith", "67890") print(library.add_book(book1)) # Output: Added Python Programming to library. print(library.add_book(book2)) # Output: Added Data Science to library. print(book1.check_out()) # Output: Python Programming checked out. print(book1.check_out()) # Output: Python Programming is already checked out. print(book1.return_book()) # Output: Python Programming returned. found_book = library.find_book("Python Programming") if found_book: print(f"Found: {found_book.title} by {found_book.author}") # Output: Found: Python Programming by John Doe
Breakdown:
- Encapsulation:
.__isbn
is private,_is_checked_out
is protected. - Classes:
Book
manages individual books,Library
manages a collection. - Methods: Clear, reusable functions for checking out/returning books and managing the library.
- Real-World Relevance: It models a practical system that is extensible for more features (e.g., users, due dates).
5. Advanced Class Features
Class vs. Instance Attributes
- Instance Attributes: Unique to each object (e.g.,
self.brand
). - Class Attributes: Shared across all instances, defined outside
__init__
.
Example:
class Student: school = "Springfield High" # Class attribute def __init__(self, name): self.name = name # Instance attribute student1 = Student("Alice") student2 = Student("Bob") print(student1.school, student2.school) # Output: Springfield High, Springfield High Student.school = "Riverside High" print(student1.school, student2.school) # Output: Riverside High, Riverside High
Static Methods and Class Methods
- Static Methods: Don’t access instance/class data, defined with
@staticmethod
. - Class Methods: Operate on the class itself, defined with
@classmethod
, takecls
as the first parameter.
Example:
class MathUtils: @staticmethod def square(num): return num * num @classmethod def describe(cls): return f"This is {cls.__name__}" print(MathUtils.square(5)) # Output: 25 print(MathUtils.describe()) # Output: This is MathUtils
Property Decorators
Properties control access to attributes, allowing getter/setter logic.
Example:
class Person: def __init__(self, name): self._name = name @property def name(self): return self._name @name.setter def name(self, value): if isinstance(value, str) and value: self._name = value else: raise ValueError("Name must be a non-empty string") person = Person("Alice") print(person.name) # Output: Alice person.name = "Bob" # Uses setter print(person.name) # Output: Bob # person.name = "" # Raises ValueError
6. Why Use Classes and OOP?
Classes and OOP shine in scenarios requiring:
- Modularity: Break complex systems into manageable components (e.g.,
Book
andLibrary
). - Reusability: Reuse code via inheritance and polymorphism.
- Maintainability: Encapsulation and abstraction make code easier to update.
- Scalability: OOP supports large projects by organizing code logically.
For example, in a web application, you might use classes to model users, products, and orders, leveraging OOP to keep the codebase clean and extensible.
7. Best Practices
- Clear Naming: Use descriptive class/method names (e.g.,
BankAccount
overBA
). - Single Responsibility: Each class should have one primary purpose.
- Use Encapsulation: Protect sensitive data with private/protected attributes.
- Document Code: Add docstrings to classes and methods.
- Avoid Overcomplication: Keep inheritance hierarchies shallow and simple.
8. Next Steps
- Practice: Extend the
Library
example (e.g., add aUser
class, due dates). - Explore: Study Python’s standard library classes (e.g.,
datetime
,collections
). - Learn Frameworks: Use OOP in frameworks like Django (models) or Flask.
- Read: Check “Fluent Python” by Luciano Ramalho for advanced OOP techniques.
Conclusion
Classes in Python are the foundation of object-oriented programming, enabling you to create modular, reusable, and maintainable code. Classes support encapsulation by defining attributes and methods, while inheritance and polymorphism enhance flexibility and code reuse.
Whether building a simple script or a complex application, mastering classes equips you to write elegant, scalable Python code. Start with small projects, experiment with the examples, and explore OOP’s power in real-world scenarios.