Comprehensive Guide to Deep Learning in Python
Introduction
Deep learning is a subset of machine learning that uses neural networks with multiple layers to model complex patterns in data. It powers applications like image recognition, natural language processing (NLP), and autonomous driving. Python, with its rich ecosystem of libraries like TensorFlow and PyTorch, is the leading language for deep learning development.
This guide provides a comprehensive introduction to deep learning using Python. You’ll learn the theory behind neural networks, explore key algorithms, and implement practical examples for tasks like image classification and text processing. By the end, you’ll be equipped to build and train deep learning models and pursue advanced applications.
1. Deep Learning Fundamentals
What is Deep Learning?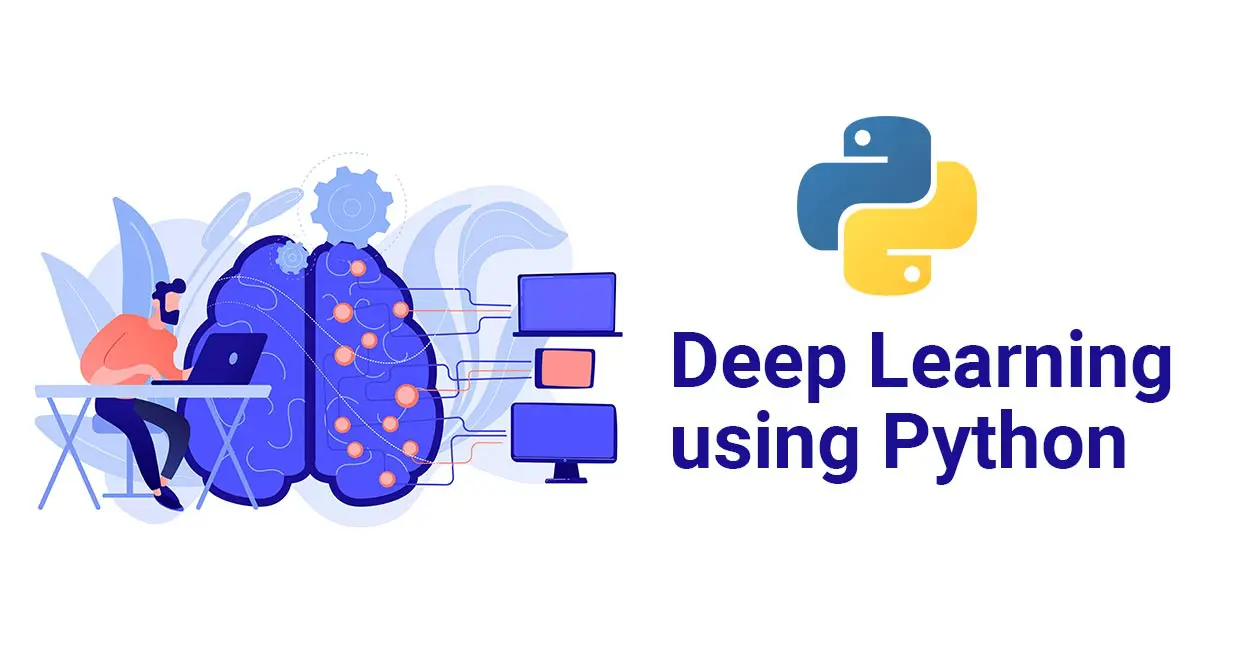
Deep learning involves training artificial neural networks with many layers (hence “deep”) to learn hierarchical representations from data. Unlike traditional machine learning, deep learning handles unstructured data (e.g., images, text) by automatically extracting features.
Analogy: Think of a neural network as a series of filters. Each layer refines the input (e.g., an image) to identify patterns, from edges in early layers to complex objects in deeper layers.
Key Components of Neural Networks
- Neurons: Basic units that compute a weighted sum of inputs, apply an activation function (e.g., ReLU, sigmoid), and output a value.
- Layers:
- Input Layer: Receives raw data (e.g., pixel values).
- Hidden Layers: Process data through transformations.
- Output Layer: Produces predictions (e.g., class probabilities).
- Weights and Biases: Parameters adjusted during training to minimize error.
- Activation Functions: Introduce non-linearity (e.g., ReLU:
max(0, x)
, sigmoid:1/(1+e^-x)
). - Loss Function: Measures prediction error (e.g., mean squared error for regression, cross-entropy for classification).
- Optimizer: Updates weights to minimize loss (e.g., Adam, SGD).
Types of Neural Networks
- Feedforward Neural Networks (FNNs): Basic networks for tabular data or simple tasks.
- Convolutional Neural Networks (CNNs): Specialized for images, using convolution layers to detect spatial patterns.
- Recurrent Neural Networks (RNNs): Designed for sequences (e.g., time series, text), with variants like LSTMs and GRUs.
- Transformers: Advanced models for NLP and vision, using attention mechanisms (e.g., BERT, GPT).
2. Python Tools for Deep Learning
Python’s ecosystem is ideal for deep learning. Key libraries include:
- TensorFlow/Keras: Scalable, production-ready framework for neural networks.
- PyTorch: Flexible, research-friendly framework, popular in academia.
- NumPy: Handles numerical operations for preprocessing.
- Pandas: Manages tabular data.
- Matplotlib/Seaborn: Visualizes training metrics (e.g., loss curves).
- scikit-learn: Provides utilities for preprocessing and evaluation.
- Hugging Face Transformers: Pre-trained models for NLP and vision.
To install these, run:
pip install tensorflow torch numpy pandas matplotlib seaborn scikit-learn transformers
Jupyter Notebook is recommended for interactive coding and visualization. If you have an NVIDIA GPU, install CUDA-compatible versions of TensorFlow/PyTorch for GPU acceleration.
3. Deep Learning Workflow
The deep learning process involves:
- Problem Definition: Identify the task (e.g., classify images, generate text).
- Data Collection: Gather and preprocess data (e.g., normalize images).
- Model Design: Choose a network architecture (e.g., CNN for images).
- Training: Optimize weights using backpropagation and an optimizer.
- Evaluation: Assess performance on a test set (e.g., accuracy, F1-score).
- Tuning: Adjust hyperparameters (e.g., learning rate, layers).
- Deployment: Integrate the model into applications (e.g., Flask API).
4. Practical Examples with Python
Let’s implement three deep learning tasks: a feedforward neural network for classification, a CNN for image classification, and a transformer for text generation. These examples use TensorFlow/Keras and PyTorch, with standard datasets for simplicity.
Example 1: Feedforward Neural Network (TensorFlow/Keras)
Classify synthetic tabular data.
import tensorflow as tf from sklearn.datasets import make_classification from sklearn.model_selection import train_test_split from sklearn.preprocessing import StandardScaler # Generate synthetic data X, y = make_classification(n_samples=1000, n_features=20, n_classes=2, random_state=42) X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=42) # Preprocess data scaler = StandardScaler() X_train = scaler.fit_transform(X_train) X_test = scaler.transform(X_test) # Build model model = tf.keras.Sequential([ tf.keras.layers.Dense(64, activation='relu', input_shape=(20,)), tf.keras.layers.Dense(32, activation='relu'), tf.keras.layers.Dense(1, activation='sigmoid') ]) # Compile model model.compile(optimizer='adam', loss='binary_crossentropy', metrics=['accuracy']) # Train model model.fit(X_train, y_train, epochs=10, batch_size=32, validation_split=0.2, verbose=1) # Evaluate loss, accuracy = model.evaluate(X_test, y_test, verbose=0) print(f"Test Accuracy: {accuracy:.2f}")
Explanation:
- Data: Synthetic dataset with 20 features and two classes.
- Model: Simple FNN with three layers (64, 32, 1 neurons).
- Training: 10 epochs, Adam optimizer, binary cross-entropy loss.
- Output: Test accuracy (e.g., ~0.90).
- Use Case: Fraud detection, customer churn prediction.
Example 2: Convolutional Neural Network (PyTorch)
Classify handwritten digits (MNIST dataset).
import torch import torch.nn as nn import torch.optim as optim from torchvision import datasets, transforms from torch.utils.data import DataLoader # Define transformations transform = transforms.Compose([transforms.ToTensor(), transforms.Normalize((0.5,), (0.5,))]) # Load MNIST data train_data = datasets.MNIST(root='./data', train=True, download=True, transform=transform) test_data = datasets.MNIST(root='./data', train=False, download=True, transform=transform) train_loader = DataLoader(train_data, batch_size=64, shuffle=True) test_loader = DataLoader(test_data, batch_size=64, shuffle=False) # Define CNN class CNN(nn.Module): def __init__(self): super(CNN, self).__init__() self.conv1 = nn.Conv2d(1, 16, kernel_size=3, padding=1) self.conv2 = nn.Conv2d(16, 32, kernel_size=3, padding=1) self.pool = nn.MaxPool2d(2, 2) self.fc1 = nn.Linear(32 * 7 * 7, 128) self.fc2 = nn.Linear(128, 10) self.relu = nn.ReLU() def forward(self, x): x = self.pool(self.relu(self.conv1(x))) x = self.pool(self.relu(self.conv2(x))) x = x.view(-1, 32 * 7 * 7) x = self.relu(self.fc1(x)) x = self.fc2(x) return x # Initialize model, loss, and optimizer model = CNN() criterion = nn.CrossEntropyLoss() optimizer = optim.Adam(model.parameters(), lr=0.001) # Train model device = torch.device("cuda" if torch.cuda.is_available() else "cpu") model.to(device) for epoch in range(5): model.train() for data, target in train_loader: data, target = data.to(device), target.to(device) optimizer.zero_grad() output = model(data) loss = criterion(output, target) loss.backward() optimizer.step() print(f"Epoch {epoch+1}, Loss: {loss.item():.4f}") # Evaluate model.eval() correct = 0 total = 0 with torch.no_grad(): for data, target in test_loader: data, target = data.to(device), target.to(device) outputs = model(data) _, predicted = torch.max(outputs.data, 1) total += target.size(0) correct += (predicted == target).sum().item() print(f"Test Accuracy: {correct / total:.2f}")
Explanation:
- Data: MNIST dataset (28×28 grayscale digit images, 10 classes).
- Model: CNN with two convolutional layers, max-pooling, and fully connected layers.
- Training: 5 epochs, Adam optimizer, cross-entropy loss.
- Output: Test accuracy (e.g., ~0.98).
- Use Case: Image classification, OCR.
Note: Requires torchvision
(pip install torchvision
). GPU usage is optional, but speeds up training.
Example 3: Text Generation with Transformers (Hugging Face)
Generate text using a pre-trained GPT-22 model.
from transformers import pipeline # Initialize text generation pipeline generator = pipeline("text-generation", model="gpt2", device=-1) # device=-1 for CPU # Generate text prompt = "Once upon a time in a distant galaxy" result = generator(prompt, max_length=50, num_return_sequences=1, truncation=True) print(result[0]['generated_text'])
Explanation:
- Model: GPT-2, a transformer for NLP tasks.
- Code: Uses Hugging Face
pipeline
for easy text generation. - Output: Generates a continuation of the prompt (e.g.,” Once upon a time in a distant galaxy, a brave explorer…”).
- Use Case: Chatbots, content generation, sentiment analysis.
Note: GPT-2 is large; ensure sufficient memory or use a smaller model (e.g., distilgpt2
) for testing.
5. Data Preprocessing
Deep learning models require clean, properly formatted data:
- Normalization: Scale inputs (e.g., images to [0,1] or [-1,1]).
- Augmentation: For images, apply random flips and rotations (e.g.,
torchvision.transforms
). - Tokenization: For text, convert to numerical tokens (e.g., Hugging Face
tokenizer
). - Batching: Group data into batches for efficient training (e.g.,
DataLoader
).
Example (Image Preprocessing):
from sklearn.preprocessing import StandardScaler import numpy as np # Normalize tabular data scaler = StandardScaler() X_scaled = scaler.fit_transform(X) # For images (already done in MNIST example via transforms.Normalize)
6. Model Evaluation and Tuning
Evaluation Metrics
- Classification: Accuracy, Precision, Recall, F1-Score, ROC-AUC.
- Regression: Mean Squared Error (MSE), Mean Absolute Error (MAE).
- Text Generation: BLEU score, human evaluation, perplexity.
Example (Plotting Loss):
import matplotlib.pyplot as plt # Assuming history from Keras model.fit history = model.fit(X_train, y_train, epochs=10, validation_split=0.2, verbose=0) plt.plot(history.history['loss'], label='Training Loss') plt.plot(history.history['val_loss'], label='Validation Loss') plt.xlabel('Epoch') plt.ylabel('Loss') plt.legend() plt.title('Training and Validation Loss') plt.show()
Hyperparameter Tuning
- Learning Rate: Start with 0.001 (Adam), adjust if loss diverges.
- Batch Size: 32 or 64 for balance; smaller for memory constraints.
- Layers/Neurons: Add layers for complex tasks, but avoid overfitting.
- Dropout: Add
Dropout
layers (e.g.,tf.keras.layers.Dropout(0.2)
) to prevent overfitting.
Example (Keras with Dropout):
model = tf.keras.Sequential([ tf.keras.layers.Dense(64, activation='relu', input_shape=(20,)), tf.keras.layers.Dropout(0.2), tf.keras.layers.Dense(32, activation='relu'), tf.keras.layers.Dropout(0.2), tf.keras.layers.Dense(1, activation='sigmoid') ])
7. Advanced Topics
Transfer Learning
Use pre-trained models to save time and data.
- Example: Fine-tune a pre-trained ResNet for image classification.
from tensorflow.keras.applications import ResNet50 from tensorflow.keras.layers import Dense, GlobalAveragePooling2D from tensorflow.keras.models import Model base_model = ResNet50(weights='imagenet', include_top=False, input_shape=(224, 224, 3)) x = GlobalAveragePooling2D()(base_model.output) x = Dense(10, activation='softmax')(x) model = Model(inputs=base_model.input, outputs=x)
Autoencoders
Unsupervised learning for dimensionality reduction or denoising.
- Example: Reconstruct MNIST images.
# Simplified autoencoder autoencoder = tf.keras.Sequential([ tf.keras.layers.Dense(128, activation='relu', input_shape=(784,)), tf.keras.layers.Dense(32, activation='relu'), tf.keras.layers.Dense(128, activation='relu'), tf.keras.layers.Dense(784, activation='sigmoid') ]) autoencoder.compile(optimizer='adam', loss='binary_crossentropy')
Reinforcement Learning
Use deep learning for decision-making (e.g., Deep Q-Networks with PyTorch).
Explainable AI
Use tools like SHAP or LIME to interpret model predictions.
8. Modern Trends (2025)
- Efficient Transformers: Models like EfficientNet, Dand andistilBERT optimize performance and size.
- AutoML: Tools like KerasTuner automate architecture search.
- Edge AI: TensorFlow Lite and PyTorch Mobile deploy models on devices.
- Federated Learning: Train models across decentralized devices (e.g., TensorFlow Federated).
- Quantum Machine Learning: Emerging libraries like PennyLane integrate quantum computing.
9. Challenges and Best Practices
- Challenges:
- Overfitting due to deep architectures.
- High computational requirements (GPU/TPU needed).
- Data scarcity for specialized tasks.
- Best Practices:
- Use data augmentation to increase the dataset size.
- Monitor training with validation metrics to avoid overfitting.
- Leverage transfer learning for small datasets.
- Save models (
model.save('model.h5')
and use checkpoints. - Optimize for production (e.g., TensorFlow Serving, ONNX).
10. Next Steps
- Practice: Apply examples to Kaggle datasets (e.g., CIFAR-10, IMDB reviews).
- Learn: Explore courses (e.g., DeepLearning.AI’s Deep Learning Specialization, Fast.ai).
- Experiment: Fine-tune Hugging Face models or build custom CNNs.
- Contribute: Join open-source projects on GitHub (e.g., TensorFlow, PyTorch).
- Stay Updated: Follow X posts from AI researchers or blogs like Towards Data Science.
11. Conclusion
Deep learning in Python unlocks robust solutions for complex problems, from image recognition to text generation. You can build, train, and deploy sophisticated neural networks with TensorFlow, PyTorch, and Hugging Face.
Start with the provided examples, experiment with real datasets, and explore advanced techniques like transfer learning and transformers. Python’s deep learning ecosystem empowers you to turn ideas into reality.