Database models in Python and Django – Databases in Django and Python
Before starting to work with the database in Django, we should get acquainted with the topic of database model in Django and examine the concept and function of the model in the database.
Databases in Django and Python, Since modern web applications typically use a back-end data storage to manage and manipulate data, it makes sense for Django to have a mechanism for automatically managing data within a database.
Django does this by providing ORM, or Object-Relational-Mapper. Django database model is a tool used to describe how data is displayed in a database.
The relationships between models are similar to the relationships between tables in a database.
Configure Django to access the database
In order for Django to manage data in the database, we must first configure the database access parameters.
These parameters are available in the settings.py file for your project and are described in the table below:
Parameter | Description |
DATABASE_ENGINE | The database engine that Django will use. Valid values of all In lower case: Postgresql: PostgreSQL that uses psycopg postgresql_psycopg2: PostgreSQL that uses psycopg2 mysql – MySQL sqlite3 – Sqlite oracle: Oracle Make sure the driver is installed for the database you want to use before attempting to use it! |
DATABASE_NAME | Database name (or, in the case of SQLite, the database file path) |
DATABASE_USER | Username to use when connecting to the database. |
DATABASE_PASSWORD | Password used when connecting to the database. |
DATABASE_HOST | The name of the host that can access the database. |
DATABASE_PORT | Port number used to connect to the database. |
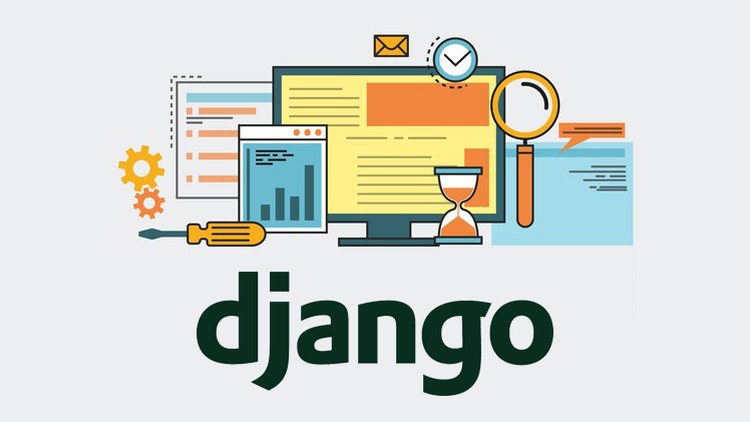
Once you have configured your parameters, you can test the availability of your database using commands below the command line (from within your project list):
1 2 3 4 5 | <span style=“font-size: 16px;”>python manage.py shell from django.db import connection cursor=connection.cursor()</span> |
Defining a database model in Django
If you look at the model.py file in a new program, you will notice that this file (by default) contains a line including:
1 | <span style=“font-size: 16px;”>from django.db import models</span> |
Django uses the django.db.models.Model subclass to represent each model. To create a new model (analog table in the database), we need to create a class that inherits from django.db.models.Model.
- A class contains a number of class attributes, and each attribute usually corresponds to a column in the table.
- Each attribute name matches the table column name.
- Each type of attribute corresponds to the type of database column. And each model field may also have one or more named parameters that indicate restrictions or other requirements related to this section.
- Django (by default) automatically adds the “id” column as the primary key to each table (unless you define a primary key for the table).
Note: If you check your Django, you will see a “Model fields” section that describes each of the model fields. These fields are created by performing field-related functions followed by optional parameters.
Understand Model fields & Options
Django model fields are divided into several categories:
- Model fields that specify the data type.
- Model fields that specify a relational model.
- These are (generally) model fields that specify one field in this model as they refer to one field in another model.
- These include: ForeignKey – ManyToManyFiled – OneToOneFiled – GenericForeignKey
GenericForeignKey is used to implement certain types of relationships that tie contexts in one application model to another (and perform a range of non-standard tasks in another model).
Each model field is also somewhat customizable using the model field options set.
There are several options that are common to all model fields and other specifics are specific types. Common options are:
Option name | Description |
null | Defaults on False, if set to True, indicate that the field may be empty. |
blank | If true, the field may be empty. The default is false. |
db_column | Database column name for use in this section. If this field is not given, Django uses the field name. |
db_index | If true, this will be an indexed part. The default value is false |
primary_key | If true, this is the key to the model. |
unique | If true, this section must be unique to the entire table. |
Table registration contract in Django
When a model becomes a table, Django always uses a specific naming convention (unless you ignore it).
Django always uses app_classname for the table name, where classname is the name of the class you specified. The letters are always written in lower case, so the names of the tables are lowercase. When using Relational Model Fields, for example, ManyToManyField, Django adds the field name to the bottom of the table to create a new table containing the relationship specified in the model.
How to create a database model in Django
Suppose you are building a website that includes an event registration system.
In this case, you probably need several tables:
- A table containing events, named events, start date, end date, start time, end time, prices, currency and event description.
- Table includes registered people, with email address, name, phone
- A table contains event logs that link registered people to events.
This table will be executed using a very large relation, so the model will create it alone.
The sample code for implementing this model is as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | <span style=“font-size: 16px;”>from django.db import models class Events(models.Model): name = models.CharField(max_length=128, help_text=‘Name of Event’) start_date = models.DateField(help_text=‘Start date of Event’) end_date = models.DateField(help_text=‘End date of Event’) start_time = models.TimeField(help_text=‘Start time of Event’) end_time = models.TimeField(help_text=‘End Time of Event’) description = models.TextField(help_text=‘Description of Event’) class People(models.Model): fname = models.CharField(max_length=64, help_text=‘First Name’) lname = models.CharField(max_length=64, help_text=‘Last Name’) email = models.EmailField(max_length=128, help_text=‘Email Address’) phone = models.CharField(max_length=16, help_text=‘Phone Number’) enrollments= models.ManyToManyField(Events)</span> |
After becoming a database structure in Django, this is the SQL code that is generated:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 | <span style=“font-size: 16px;”>BEGIN; CREATE TABLE “demoapp_events” ( “id” integer NOT NULL PRIMARY KEY, “name” varchar(128) NOT NULL, “start_date” date NOT NULL, “end_date” date NOT NULL, “start_time” time NOT NULL, “end_time” time NOT NULL, “description” text NOT NULL ) ; CREATE TABLE “demoapp_people” ( “id” integer NOT NULL PRIMARY KEY, “fname” varchar(64) NOT NULL, “lname” varchar(64) NOT NULL, “email” varchar(128) NOT NULL, “phone” varchar(16) NOT NULL ) ; CREATE TABLE “demoapp_people_enrollments” ( “id” integer NOT NULL PRIMARY KEY, “people_id” integer NOT NULL REFERENCES “demoapp_people” (“id”), “events_id” integer NOT NULL REFERENCES “demoapp_events” (“id”), UNIQUE (“people_id”, “events_id”) ) ; COMMIT;</span> |
Notice how Django automatically creates extra tables to implement multiple relationships.
Model validation in Django
Using the “validation” command with manage.py, you can verify your models to use the correct syntax and packages.
This command validates all project models, not just a single program. As a result, it is usually advisable to add a program and validate it, as opposed to adding and validating the program all at once.
Correct errors and continue validation until command 0 returns an error.
1 | <span style=“font-size: 16px;”>python manage.py validate</span> |
Generate and execute SQL
After creating the program, you can check the SQL that your model generates.
The command is to create SQL code to check sqlall. Once again, use the mange.py sqlall command, followed by the program name to generate SQL code for checking.
When you are ready to create tables in your database, you can issue the following command:
1 | <span style=“font-size: 16px;”>python manage.py syncdb</span> |
This command connects to the database and creates tables for all the programs that are connected to the project. If a table already exists, Django confirms that it contains at least the columns defined by the program. If the table has extra columns (or there are extra tables in the database), Django does not return an error. But if the table does not have the required column or type, Django returns an error.
1 | <span style=“font-size: 16px;”>python manage.py sqlall demoapp</span> |
Add data to the model in Django
Once you have created the model, it is relatively easy to add data to it: first create a new object for the model you want to add to that data.
The constructor must pass all the required background data in name / value pairs.
Once created, you can save it to the database using the .save () method. This technique works for all models, adding data is just a creation for the model and saving it.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 | <span style=“font-size: 16px;”>from demoapp.models import Events from datetime import date event = Events(name=‘Introduction to Python’, start_date=date(2007,01,01), end_date=date(2007,01,04), start_time=’09:00:00′, end_time=’17:00:00′, description=‘This is a Python training event’) event.save()</span> |
Simple data retrieval using a model
Now that we know how to put data into a model and update it, let’s talk a little bit about retrieving data from a model.
There are several different ways we can use a simple model to retrieve data, such as the one we created earlier:
You can use the all () method to retrieve all rows from a specific table. The all () method returns QuerySet like most data return methods. If repeated, QuerySet returns instances of the model object, and each instance contains data from that record. To update the database, each object can be modified and saved.
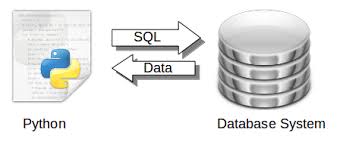
tip:
Each model in Django is associated with a Manager (the manager is referenced by the .object attribute of the model). Manager is an interface through which query operations are performed on Django models. The manager itself provides a set of methods for communicating with the database.
1 2 3 4 5 | <span style=“font-size: 16px;”>data = Events.objects.all() for e in data: print e.name, ‘starts on’, e.start_date</span> |
Delete records in Django
Your .delete () method is used to delete records from the data model. This method may also run in QuerySet to remove all records referenced by QuerySet.
Delete all records from the Event model
1 2 3 4 5 | <span style=“font-size: 16px;”>data = Events.objects.all() for e in data: e.delete()</span> |
Delete all records from the Event model
1 | <span style=“font-size: 16px;”>data = Events.objects.all().delete()</span> |
Remove all past events from the Event model
1 2 3 4 5 | <span style=“font-size: 16px;”>data = Events.objects.all() data = data.filter(start_date__lte=date.today()) data.delete()</span> |
Enable management interface Using Django management interface (Django management interface)
The management interface is designed to provide an easy way to manage and manipulate the data stored in the model. For a specific model to appear in the admin interface, you must first create admin.py in your application.
The admin.py file contains the display of your models in the admin interface (ModelAdmin).
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | <span style=“font-size: 16px;”>from django.contrib import admin from demo.demoapp.models import * class EventsAdmin(admin.ModelAdmin): list_display=(‘name’,‘start_date’) admin.site.register(Events, EventsAdmin) class PeopleAdmin(admin.ModelAdmin): list_display=(‘lname’,‘fname’) admin.site.register(People, PeopleAdmin)</span> |
To display it, you must register it in the Admin section of the site. Once you’ve updated your models, syncdb to make sure the model syncs with the database layout. To activate the admin interface, you must add a URL to it to urls.py:
admin.py:
This file contains a display of People and Events models in the admin interface. When this item is added, these objects will appear in that interface.
1 2 3 4 5 | <span style=“font-size: 16px;”>from django.contrib import admin admin.autodiscover() urlpatterns += patterns (”,(r‘^admin/’, include(admin.site.urls)))</span> |
You should also add django.contrib.admin in settings.py to INSTALLED_APPS.
Finally, run syncdb to create the required admin components. Finally, ModelAdmin has a number of features that may be configured to control how the admin site is displayed.
For example, setting the list_display property allows us to list the columns displayed on the list change manager page.