Introduction
Network security protects computer networks from unauthorized access, data breaches, and malicious activities. With cyber threats evolving rapidly, Python’s versatility and rich ecosystem make it an excellent tool for building and automating security solutions.
From scanning for vulnerabilities to encrypting sensitive data, Python empowers developers and security professionals to strengthen network defenses.
This guide provides a comprehensive overview of using Python to enhance network security. You’ll learn key concepts, explore Python libraries, and implement practical solutions like vulnerability scanning, secure communication, and intrusion detection. By the end, you’ll have the tools to safeguard networks effectively.
1. Network Security Fundamentals
What is Network Security?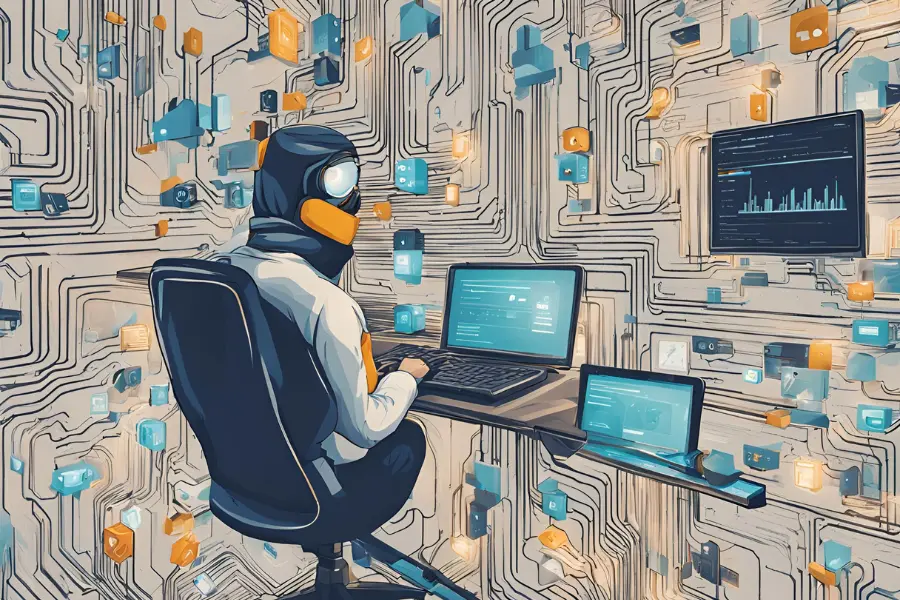
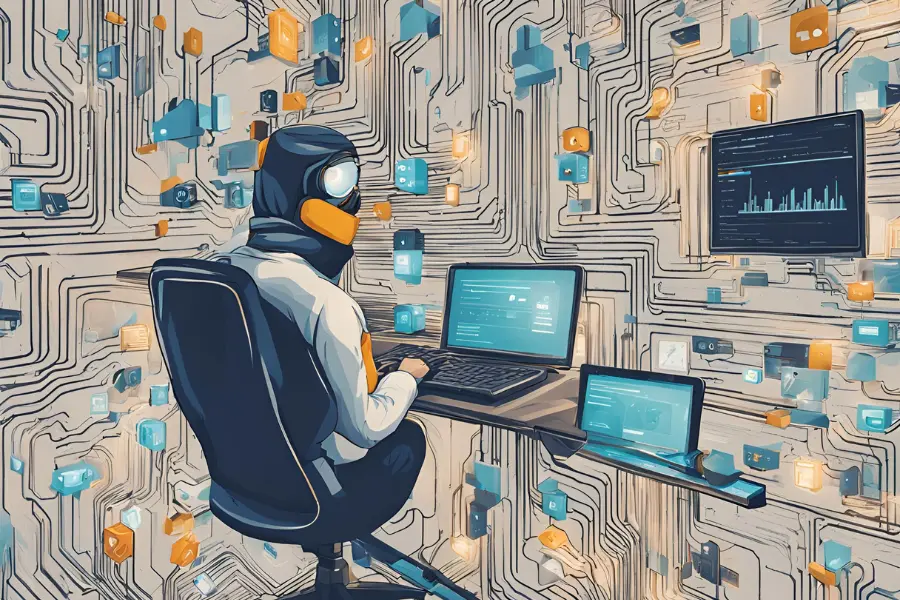
Network security is the process of securing a network’s infrastructure, data, and services against threats such as hacking, malware, and denial-of-service (DoS) attacks. Think of a network as a fortress: to protect it, you need strong walls (firewalls), guards (intrusion detection), and secure gates (encryption).
Core Principles
- Confidentiality: Ensure data is only accessible to authorized users (e.g., encryption).
- Integrity: Protect data from tampering (e.g., hashing).
- Availability: Keep services running despite attacks (e.g., DoS mitigation).
- Authentication: Verify user/device identities (e.g., secure logins).
- Authorization: Control access levels (e.g., role-based access).
Common Threats
- Malware: Malicious software (e.g., viruses, ransomware).
- Phishing: Social engineering to steal credentials.
- Packet Sniffing: Intercepting network traffic to steal data.
- Vulnerabilities: Exploitable weaknesses in software or configurations.
- DoS Attacks: Overloading systems to disrupt services.
Python’s Role
Python simplifies security tasks through automation, analysis, and integration. It’s used for:
- Scanning networks for vulnerabilities.
- Encrypting communications.
- Monitoring traffic for suspicious activity.
- Automating security workflows.
2. Python Tools for Network Security
Python offers powerful libraries for security tasks:
- scapy: Packet manipulation and network scanning.
- Cryptography: Encryption, decryption, and hashing.
- paramiko: Secure SSH connections for remote administration.
- Socket: Low-level network programming (e.g., port scanning).
- Requests: Testing web application security.
- pyOpenSSL: SSL/TLS certificate management.
- Pandas/NumPy: Analyzing security logs.
- scikit-learn: Machine learning for anomaly detection.
To install these, run:
pip install scapy cryptography paramiko requests pyOpenSSL pandas numpy scikit-learn
Note: Some tasks (e.g., scanning) require root privileges on Linux/macOS or administrative rights on Windows. Always test in a controlled environment and comply with legal/ethical guidelines.
3. Practical Examples with Python
Let’s implement four network security tasks: port scanning, data encryption, secure SSH connections, and intrusion detection. These examples are simplified for learning and assume you have the required libraries installed.
Example 1: Port Scanning with socket
Scan a target host for open ports to identify potential vulnerabilities.
import socket import threading from queue import Queue def scan_port(host, port, result_queue): sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) sock.settimeout(1) try: result = sock.connect_ex((host, port)) if result == 0: result_queue.put(port) except Exception: pass finally: sock.close() def scan_ports(host, start_port, end_port): print(f"Scanning {host} from port {start_port} to {end_port}...") result_queue = Queue() threads = [] for port in range(start_port, end_port + 1): thread = threading.Thread(target=scan_port, args=(host, port, result_queue)) threads.append(thread) thread.start() for thread in threads: thread.join() open_ports = [] while not result_queue.empty(): open_ports.append(result_queue.get()) return sorted(open_ports) # Usage host = "127.0.0.1" # Replace with target IP (use with permission) open_ports = scan_ports(host, 1, 100) print(f"Open ports: {open_ports}")
Explanation:
- Purpose: Identifies open ports (e.g., 80 for HTTP) that could be exploited.
- Code: Uses
socket
to attempt connections on ports 1–100, leveraging threading for speed. - Output: Lists open ports (e.g.,
[22, 80]
if SSH and HTTP run). - Ethics: Only scan networks you own or have explicit permission to test.
Warning: Unauthorized scanning may violate laws or terms of service. Use responsibly.
Example 2: Data Encryption with cryptography
Encrypt sensitive data to ensure confidentiality during transmission.
from cryptography.fernet import Fernet # Generate and save a key key = Fernet.generate_key() with open("secret.key", "wb") as key_file: key_file.write(key) # Encrypt data fernet = Fernet(key) message = "Sensitive data: credit_card=1234-5678-9012-3456" encrypted = fernet.encrypt(message.encode()) print(f"Encrypted: {encrypted}") # Decrypt data decrypted = fernet.decrypt(encrypted).decode() print(f"Decrypted: {decrypted}")
Explanation:
- Purpose: Protects data from interception (e.g., packet sniffing).
- Code: Uses
Fernet
(symmetric encryption) to encrypt and decrypt a message. - Output: Shows encrypted bytes and the original message after decryption.
- Use Case: Securely transmit sensitive data over a network.
Security Note: Store keys securely (e.g., in a vault) and never hardcode them.
Example 3: Secure SSH with paramiko
Automate secure remote server administration.
import paramiko def ssh_connect(host, username, password, command): try: # Initialize SSH client client = paramiko.SSHClient() client.set_missing_host_key_policy(paramiko.AutoAddPolicy()) # Connect client.connect(host, username=username, password=password, timeout=5) # Execute command stdin, stdout, stderr = client.exec_command(command) output = stdout.read().decode() error = stderr.read().decode() if error: return f"Error: {error}" return f"Output: {output}" except Exception as e: return f"Connection failed: {str(e)}" finally: client.close() # Usage host = "192.168.1.100" # Replace with target IP username = "user" # Replace with SSH username password = "pass" # Replace with SSH password command = "uptime" # Example command print(ssh_connect(host, username, password, command))
Explanation:
- Purpose: Securely manage remote servers (e.g., check system status).
- Code: Used
paramiko
to establish an SSH connection and run a command. - Output: Displays command output (e.g., server uptime) or errors.
- Security: Use key-based authentication in production instead of passwords.
Warning: Hardcoding credentials is insecure. Use SSH keys or a secrets manager.
Example 4: Intrusion Detection with scapy
Detect suspicious network traffic (e.g., port scans).
from scapy.all import sniff, IP, TCP import time def detect_port_scan(packet): if packet.haslayer(TCP) and packet.haslayer(IP): flags = packet[TCP].flags # SYN packets are common in port scans if flags == "S": src_ip = packet[IP].src dst_port = packet[TCP].dport print(f"Possible port scan from {src_ip} to port {dst_port}") def start_sniffing(interface="eth0", duration=10): print(f"Sniffing on {interface} for {duration} seconds...") sniff(iface=interface, prn=detect_port_scan, timeout=duration) # Usage (requires root privileges) # start_sniffing(interface="eth0", duration=10)
Explanation:
- Purpose: Identifies potential attacks by analyzing network packets.
- Code: Uses
scapy
to sniff TCP SYN packets, a hallmark of port scans. - Output: Logs suspicious activity (e.g., source IP and target port).
- Requirements: Run as root/admin (e.g.,
sudo python script.py
) and specify your network interface.
Note: Uncomment the start_sniffing
call to test in a controlled environment. Replace "eth0"
with your interface (e.g., wlan0
check with ifconfig
or ip link
).
4. Best Practices for Network Security with Python
- Secure Coding:
- Validate inputs to prevent injection attacks.
- Use secure libraries (e.g.,
cryptography
over custom encryption). - Avoid hardcoding sensitive data (e.g., keys, passwords).
- Network Hygiene:
- Use firewalls to restrict unnecessary ports.
- Enable HTTPS/TLS for web communications.
- Regularly update software to patch vulnerabilities.
- Monitoring and Logging:
- Log security events for analysis (e.g., with
logging
module). - Use SIEM tools or Python scripts to aggregate logs.
- Log security events for analysis (e.g., with
- Ethical Use:
- Obtain permission before scanning or testing networks.
- Comply with laws (e.g., CFAA in the US) and organizational policies.
- Performance:
- Optimize scripts for large networks (e.g., use threading, asyncio).
- Test scripts in a lab environment before deployment.
5. Advanced Topics and Trends (2025)
Advanced Techniques
- Machine Learning for Anomaly Detection:
Usescikit-learn
to detect unusual patterns in network traffic.from sklearn.ensemble import IsolationForest import pandas as pd # Synthetic traffic data data = pd.DataFrame({ 'packet_size': [100, 150, 200, 5000, 120], 'interval': [1, 1.2, 0.9, 0.1, 1.1] }) model = IsolationForest(contamination=0.2, random_state=42) data['anomaly'] = model.fit_predict(data) print(data[data['anomaly'] == -1]) # Anomalies
- Zero-Trust Architecture:
Implement continuous authentication with Python (e.g., verify devices via API tokens). - Automated Penetration Testing:
Usemetasploit
or custom Python scripts to simulate attacks.
Modern Trends
- AI-Driven Security: Machine learning enhances threat detection (e.g., predicting DoS attacks).
- Cloud-Native Security: Python scripts secure AWS/GCP networks (e.g., boto3 for AWS).
- IoT Security: Protect devices with Python-based monitoring (e.g., Raspberry Pi scripts).
- Post-Quantum Cryptography: Libraries like
cryptography
are adopting quantum-resistant algorithms.
6. Challenges and Solutions
- Challenges:
- False positives in intrusion detection.
- Scalability for large networks.
- Keeping up with evolving threats.
- Solutions:
- Tune detection thresholds (e.g.,
contamination
in Isolation Forest). - Use distributed computing (e.g., Dask) for big data.
- Subscribe to threat intelligence feeds and update scripts.
- Tune detection thresholds (e.g.,
7. Next Steps
- Practice: Test the examples in a lab environment (e.g., VirtualBox with Kali Linux).
- Learn: Explore courses (e.g., Coursera’s Cybersecurity Specialization, TryHackMe).
- Experiment: Build a Python-based firewall or log analyzer.
- Contribute: Join open-source security projects (e.g., Scapy, OWASP).
- Stay Updated: Follow blogs like Krebs on Security or X posts from cybersecurity experts.
8. Conclusion
Python is a powerful ally in enhancing network security, offering tools to scan vulnerabilities, encrypt data, automate tasks, and detect threats. By mastering libraries like scapy
, cryptography
, and paramikoYou can
Build robust defenses against cyber threats. Start with the provided examples, practice ethically, and explore advanced techniques to stay ahead in the ever-evolving field of network security. With Python, you’re equipped to protect networks effectively and efficiently.